pandas的concat
时间: 2023-10-27 07:49:42 浏览: 68
函数有哪些参数?
pandas的concat函数有三个主要参数,分别是objs、axis和join。其中objs是要连接的pandas对象的序列,可以是DataFrame或Series。axis是连接的轴,可以是0或1,表示连接的方向是行还是列。join是连接的方式,可以是inner或outer,表示要连接的数据集的索引是否要求交集或并集。还有其他一些可选参数,如keys、levels、names等,可以对连接后的结果进行更进一步的处理。
相关问题
pandas concat
Pandas concat is a function that is used to concatenate two or more pandas dataframes or series along a specified axis. Concatenation is a process of combining data from different sources into a single dataset. The concat function can be used to combine dataframes or series with similar or different column names and index values.
Syntax:
```
pd.concat(objs, axis=0, join='outer', ignore_index=False, keys=None, levels=None, names=None, verify_integrity=False, sort=False, copy=True)
```
Parameters:
- objs: A sequence or mapping of Series or DataFrame objects that will be concatenated.
- axis: The axis to concatenate along (0 or 1).
- join: The type of join to perform. Acceptable values are ‘inner’, ‘outer’, ‘left’, and ‘right’. The default value is ‘outer’.
- ignore_index: A boolean value indicating whether to reset the index of the concatenated DataFrame. The default value is False.
- keys: A sequence of labels to use as the new index levels. This parameter is only valid when concatenating along the columns (axis=1).
- levels: A sequence of sequences that define the new index levels. This parameter is only valid when concatenating along the columns (axis=1).
- names: A sequence of names for the new index levels. This parameter is only valid when concatenating along the columns (axis=1).
- verify_integrity: A boolean value indicating whether to check for duplicate index values. The default value is False.
- sort: A boolean value indicating whether to sort the resulting DataFrame by the column names. The default value is False.
- copy: A boolean value indicating whether to copy the data. The default value is True.
Example:
```
import pandas as pd
# create two dataframes
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3'],
'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']})
df2 = pd.DataFrame({'A': ['A4', 'A5', 'A6', 'A7'],
'B': ['B4', 'B5', 'B6', 'B7'],
'C': ['C4', 'C5', 'C6', 'C7'],
'D': ['D4', 'D5', 'D6', 'D7']})
# concatenate dataframes
result = pd.concat([df1, df2])
print(result)
```
Output:
```
A B C D
0 A0 B0 C0 D0
1 A1 B1 C1 D1
2 A2 B2 C2 D2
3 A3 B3 C3 D3
0 A4 B4 C4 D4
1 A5 B5 C5 D5
2 A6 B6 C6 D6
3 A7 B7 C7 D7
```
pandas concat函数
pandas concat函数是将两个或多个数据框或系列沿指定轴进行连接的函数。它可以按行或列进行连接,可以在连接时指定连接方式、连接键、是否保留索引等选项。具体语法如下:
```python
pandas.concat(objs, axis=0, join='outer', ignore_index=False, keys=None, sort=False, verify_integrity=False, copy=True)
```
其中,参数含义如下:
- objs:要连接的数据框或系列的列表或字典。
- axis:指定连接的轴,0表示按行连接,1表示按列连接,默认为0。
- join:指定连接方式,可以取值'outer'、'inner'、'left'、'right',分别表示外连接、内连接、左连接、右连接,默认为'outer'。
- ignore_index:是否忽略原来的索引,重新生成索引,默认为False。
- keys:将连接的数据框或系列加上一个多级索引,可以是列表、元组或数组,默认为None。
- sort:是否对连接后的数据进行排序,默认为False。
- verify_integrity:是否检查连接后的数据是否有重复索引,默认为False。
- copy:是否复制数据,默认为True。
示例:
```python
import pandas as pd
# 创建两个数据框
df1 = pd.DataFrame({'A': [1, 2], 'B': [3, 4]})
df2 = pd.DataFrame({'A': [5, 6], 'B': [7, 8]})
# 按行连接
df_concat = pd.concat([df1, df2])
print(df_concat)
# 按列连接
df_concat = pd.concat([df1, df2], axis=1)
print(df_concat)
# 指定连接键
df1 = pd.DataFrame({'A': [1, 2], 'B': [3, 4], 'key': ['K0', 'K1']})
df2 = pd.DataFrame({'C': [5, 6], 'D': [7, 8], 'key': ['K0', 'K1']})
df_concat = pd.concat([df1, df2], keys=['df1', 'df2'], join='inner', ignore_index=True)
print(df_concat)
```
输出结果:
```
A B
0 1 3
1 2 4
0 5 7
1 6 8
A B A B
0 1 3 5 7
1 2 4 6 8
key
0 K0
1 K1
2 K0
3 K1
```
阅读全文
相关推荐
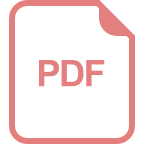
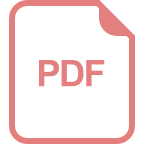
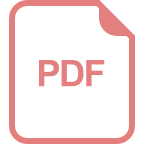













