实现论坛管理,1、在mysql中创建论坛表,包含留言时间、留言IP地址、留言内容,2、在Servlet程序中实现发表留言、留言显示、删除留言
时间: 2024-05-16 16:12:00 浏览: 11
1. 在MySQL中创建论坛表
```mysql
CREATE TABLE forum (
id INT(11) NOT NULL AUTO_INCREMENT,
message_time DATETIME NOT NULL,
message_ip VARCHAR(50) NOT NULL,
message_content VARCHAR(500) NOT NULL,
PRIMARY KEY (id)
);
```
2. 在Servlet程序中实现发表留言、留言显示、删除留言
```java
// 发表留言
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String content = request.getParameter("content");
String ip = request.getRemoteAddr();
LocalDateTime now = LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String formattedDateTime = now.format(formatter);
try {
Class.forName("com.mysql.jdbc.Driver");
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
PreparedStatement ps = con.prepareStatement("INSERT INTO forum (message_time, message_ip, message_content) VALUES (?, ?, ?)");
ps.setString(1, formattedDateTime);
ps.setString(2, ip);
ps.setString(3, content);
ps.executeUpdate();
con.close();
} catch (Exception e) {
e.printStackTrace();
}
response.sendRedirect(request.getContextPath() + "/index.jsp");
}
// 留言显示
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
List<Message> messages = new ArrayList<Message>();
try {
Class.forName("com.mysql.jdbc.Driver");
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
PreparedStatement ps = con.prepareStatement("SELECT * FROM forum ORDER BY message_time DESC");
ResultSet rs = ps.executeQuery();
while (rs.next()) {
int id = rs.getInt("id");
LocalDateTime time = LocalDateTime.parse(rs.getString("message_time"), DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"));
String ip = rs.getString("message_ip");
String content = rs.getString("message_content");
Message message = new Message(id, time, ip, content);
messages.add(message);
}
con.close();
} catch (Exception e) {
e.printStackTrace();
}
request.setAttribute("messages", messages);
request.getRequestDispatcher("/index.jsp").forward(request, response);
}
// 删除留言
protected void doDelete(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
try {
Class.forName("com.mysql.jdbc.Driver");
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/forum", "root", "password");
PreparedStatement ps = con.prepareStatement("DELETE FROM forum WHERE id = ?");
ps.setInt(1, id);
ps.executeUpdate();
con.close();
} catch (Exception e) {
e.printStackTrace();
}
response.setStatus(HttpServletResponse.SC_NO_CONTENT);
}
```
其中,`Message`类表示一条留言,包含`id`、`time`、`ip`和`content`四个字段。在留言显示时,将查询到的所有留言存储在一个`List<Message>`中,并将其传递给`index.jsp`页面进行展示。在删除留言时,根据留言的`id`进行删除操作,并返回`HttpServletResponse.SC_NO_CONTENT`状态码表示删除成功。
相关推荐
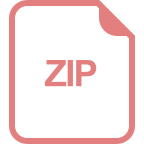
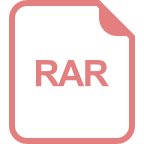
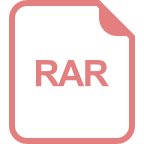








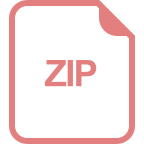
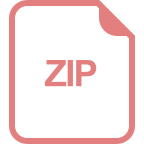
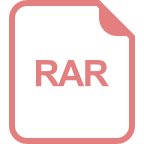
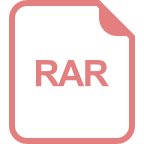
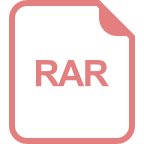
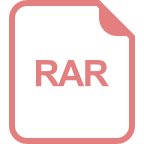
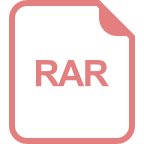
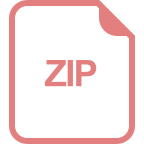