python实现语音流断句
时间: 2023-11-14 18:16:56 浏览: 47
要实现语音流断句,可以借助VAD(Voice Activity Detection)技术,即语音活动检测技术,将语音流划分为不同的语音段。
在Python中,可以使用PyAudio库来录制语音流,并使用webrtcvad库来进行VAD。具体的实现步骤如下:
1. 安装PyAudio和webrtcvad库:
```
pip install pyaudio webrtcvad
```
2. 导入库:
```python
import pyaudio
import webrtcvad
```
3. 初始化PyAudio:
```python
p = pyaudio.PyAudio()
```
4. 设置音频流参数:
```python
FORMAT = pyaudio.paInt16
RATE = 16000
CHANNELS = 1
CHUNK_DURATION_MS = 30 # 每个语音段的长度
PADDING_DURATION_MS = 150 # 语音段之间的间隔
CHUNK_SIZE = int(RATE * CHUNK_DURATION_MS / 1000) # 每个语音段的大小
PADDING_SIZE = int(RATE * PADDING_DURATION_MS / 1000) # 语音段之间的间隔大小
```
5. 定义VAD参数:
```python
vad = webrtcvad.Vad(3) # 设置VAD的敏感度,1-3依次增加
```
6. 定义断句函数:
```python
def vad_collector(sample_rate, padding_ms, vad, frames):
num_padding_frames = int(padding_ms / 30)
ring_buffer = collections.deque(maxlen=num_padding_frames)
triggered = False
voiced_frames = []
for frame in frames:
is_speech = vad.is_speech(frame.bytes, sample_rate)
sys.stdout.write('1' if is_speech else '0')
if not triggered:
ring_buffer.append(frame)
num_voiced = len([f for f in ring_buffer if vad.is_speech(f.bytes, sample_rate)])
if num_voiced > 0.9 * ring_buffer.maxlen:
triggered = True
voiced_frames.extend(ring_buffer)
ring_buffer.clear()
else:
voiced_frames.append(frame)
ring_buffer.append(frame)
num_unvoiced = len([f for f in ring_buffer if not vad.is_speech(f.bytes, sample_rate)])
if num_unvoiced > 0.9 * ring_buffer.maxlen:
triggered = False
yield b''.join([f.bytes for f in voiced_frames])
ring_buffer.clear()
voiced_frames = []
if triggered:
yield b''.join([f.bytes for f in voiced_frames])
```
7. 录制并断句:
```python
stream = p.open(format=FORMAT, channels=CHANNELS, rate=RATE, input=True, frames_per_buffer=CHUNK_SIZE)
print("开始录音")
frames = []
for i in range(int(RATE / CHUNK_SIZE * 30)): # 录制30秒
data = stream.read(CHUNK_SIZE)
frames.append(data)
print("录音结束")
stream.stop_stream()
stream.close()
p.terminate()
# 对录制的语音流进行断句
chunks = list(vad_collector(RATE, PADDING_DURATION_MS, vad, Frame(idx, data) for idx, data in enumerate(frames)))
```
这样,chunks中就存储了断句后的语音段。
相关推荐
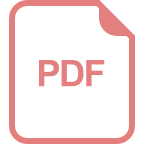
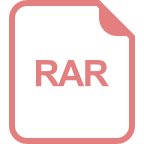
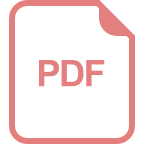











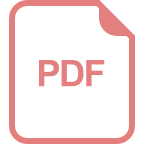
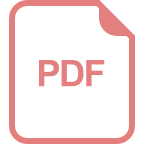