用Java写一个量化交易策略,双均线策略
时间: 2023-10-10 17:09:24 浏览: 49
好的,双均线策略是一种经典的量化交易策略,下面是用Java实现的代码:
```java
import java.util.ArrayList;
import java.util.List;
public class DualMovingAverageStrategy {
// 定义双均线策略的参数
private int shortPeriod;
private int longPeriod;
private List<Double> shortMA = new ArrayList<>();
private List<Double> longMA = new ArrayList<>();
public DualMovingAverageStrategy(int shortPeriod, int longPeriod) {
this.shortPeriod = shortPeriod;
this.longPeriod = longPeriod;
}
/**
* 计算移动平均值
* @param priceList 价格列表
* @param period 周期
* @return 移动平均值
*/
private double calculateMA(List<Double> priceList, int period) {
double sum = 0;
for (int i = priceList.size() - period; i < priceList.size(); i++) {
sum += priceList.get(i);
}
return sum / period;
}
/**
* 更新双均线策略
* @param price 最新的股票价格
*/
public void update(double price) {
// 添加最新的价格到价格列表中
shortMA.add(price);
longMA.add(price);
// 如果价格列表的长度大于均线的周期,则计算均线
if (shortMA.size() > shortPeriod) {
shortMA.remove(0);
longMA.remove(0);
double shortAvg = calculateMA(shortMA, shortPeriod);
double longAvg = calculateMA(longMA, longPeriod);
// 判断是否出现金叉或死叉
if (shortAvg > longAvg) {
System.out.println("金叉出现,买入股票!");
} else if (shortAvg < longAvg) {
System.out.println("死叉出现,卖出股票!");
}
}
}
}
```
使用方法:
```java
DualMovingAverageStrategy strategy = new DualMovingAverageStrategy(5, 10); // 创建双均线策略,短周期为5,长周期为10
strategy.update(10); // 更新策略,传入最新的价格
strategy.update(12);
strategy.update(15);
strategy.update(14);
strategy.update(16);
strategy.update(18); // 出现金叉,买入股票!
strategy.update(20);
strategy.update(19);
strategy.update(17); // 出现死叉,卖出股票!
```
以上就是用Java实现双均线策略的代码,希望对你有帮助!
相关推荐
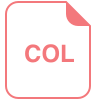
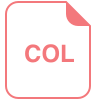
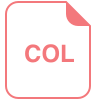
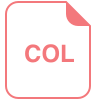
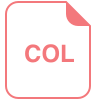







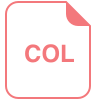
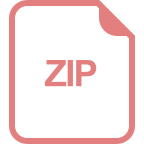
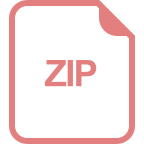
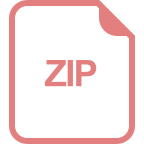
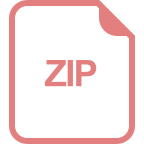