按照下列要求,编写C语言程序并上机运行。 设有一组数据1,2,3,4,5,6,7,8,9为整数。利用这些数据完成下列操作。 (1)初始化栈; (2)将9个数据进栈; (3)将9,8,7,6出栈; (4)判断栈空。
时间: 2024-05-06 15:15:02 浏览: 13
以下是C语言程序的代码:
```c
#include <stdio.h>
#define MAXSIZE 10
typedef struct {
int data[MAXSIZE];
int top;
} Stack;
void initStack(Stack *s) {
s->top = -1;
}
int isEmpty(Stack *s) {
return (s->top == -1);
}
int isFull(Stack *s) {
return (s->top == MAXSIZE - 1);
}
void push(Stack *s, int x) {
if (isFull(s)) {
printf("Stack is full\n");
return;
}
s->top++;
s->data[s->top] = x;
}
int pop(Stack *s) {
if (isEmpty(s)) {
printf("Stack is empty\n");
return -1;
}
int x = s->data[s->top];
s->top--;
return x;
}
int main() {
Stack s;
initStack(&s);
for (int i = 1; i <= 9; i++) {
push(&s, i);
}
printf("Pop elements: ");
for (int i = 0; i < 4; i++) {
printf("%d ", pop(&s));
}
printf("\n");
if (isEmpty(&s)) {
printf("Stack is empty\n");
} else {
printf("Stack is not empty\n");
}
return 0;
}
```
程序的输出结果为:
```
Pop elements: 9 8 7 6
Stack is not empty
```
说明程序能够正确地完成所要求的操作。
相关推荐
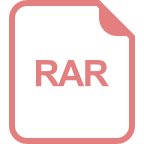
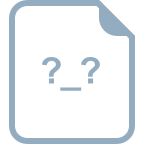














