编写程序输入月份和日期,给出对应的星座
时间: 2023-05-31 14:19:51 浏览: 651
### 回答1:
好的,以下是程序的代码:
```python
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
if month == 1:
if day <= 19:
print("摩羯座")
else:
print("水瓶座")
elif month == 2:
if day <= 18:
print("水瓶座")
else:
print("双鱼座")
elif month == 3:
if day <= 20:
print("双鱼座")
else:
print("白羊座")
elif month == 4:
if day <= 19:
print("白羊座")
else:
print("金牛座")
elif month == 5:
if day <= 20:
print("金牛座")
else:
print("双子座")
elif month == 6:
if day <= 21:
print("双子座")
else:
print("巨蟹座")
elif month == 7:
if day <= 22:
print("巨蟹座")
else:
print("狮子座")
elif month == 8:
if day <= 22:
print("狮子座")
else:
print("处女座")
elif month == 9:
if day <= 22:
print("处女座")
else:
print("天秤座")
elif month == 10:
if day <= 23:
print("天秤座")
else:
print("天蝎座")
elif month == 11:
if day <= 22:
print("天蝎座")
else:
print("射手座")
elif month == 12:
if day <= 21:
print("射手座")
else:
print("摩羯座")
else:
print("输入的月份不正确,请重新输入!")
```
这个程序可以根据输入的月份和日期,输出对应的星座。例如,如果输入的是3月15日,程序会输出“双鱼座”。
### 回答2:
编写程序输入月份和日期,给出对应的星座,需要使用到条件语句和比较运算符。
首先,我们需要了解星座的划分规则。通常我们认为星座是按照太阳在黄道上的位置划分的,每个星座对应着一段黄道上的区间。例如白羊座对应着3月21日到4月19日这段时间,双子座对应着5月21日到6月21日这段时间。
根据这些信息,我们可以编写程序输入月份和日期,然后根据各星座的黄道区间,判断输入的日期是否在某个星座范围内。为了便于判断,可以将每个星座的起始日期保存在一个列表中。然后使用条件语句和比较运算符来判断输入的日期属于哪个星座。
下面给出一个Python的示例程序:
```python
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
constellations = ['摩羯座', '水瓶座', '双鱼座', '白羊座', '金牛座', '双子座', '巨蟹座', '狮子座', '处女座', '天秤座', '天蝎座', '射手座', '摩羯座']
start_dates = [20, 19, 21, 20, 21, 21, 22, 23, 23, 23, 22, 22]
if day < start_dates[month - 1]:
constellation = constellations[(month - 2) % 12]
else:
constellation = constellations[(month - 1) % 12]
print("您的星座是:", constellation)
```
在上面的程序中,我们首先输入了月份和日期。然后定义了一个常量列表`constellations`,保存了各个星座的名称,以及一个起始日期列表`start_dates`,保存了各个星座的起始日期,例如白羊座的起始日期为3月21日,就保存在`start_dates`列表的第4个元素中。
接着,使用if语句和比较运算符,判断输入的日期是否在当前月份的星座范围内。需要注意的是,如果输入的日期比该星座的起始日期还早,那么应该属于上一个星座。所以我们使用了取余数的操作来判断上一个星座的索引。
最后,输出判断结果,显示对应的星座。
当然,这只是一个简单的示例程序,还有很多值得优化和改进的地方。例如可以对输入进行合法性检查,判断输入的月份和日期是否在正确的范围内;也可以将程序封装成一个函数,方便调用和复用。
### 回答3:
星座可以根据每年月份的不同划分,每个星座都有其对应的时间段,因此我们可以编写程序输入月份和日期来给出对应的星座。
首先,我们需要定义每个星座对应的时间段。例如,白羊座的时间段是3月21日至4月19日,金牛座的时间段是4月20日至5月20日等等。根据这些定义好的时间段,我们可以使用if语句来判断输入的日期和月份是否符合某个星座的时间段。
以下是一个Python程序来实现该功能:
```
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
if (month == 1 and day >= 20) or (month == 2 and day <= 18):
print("你的星座是水瓶座")
elif (month == 2 and day >= 19) or (month == 3 and day <= 20):
print("你的星座是双鱼座")
elif (month == 3 and day >= 21) or (month == 4 and day <= 19):
print("你的星座是白羊座")
elif (month == 4 and day >= 20) or (month == 5 and day <= 20):
print("你的星座是金牛座")
elif (month == 5 and day >= 21) or (month == 6 and day <= 21):
print("你的星座是双子座")
elif (month == 6 and day >= 22) or (month == 7 and day <= 22):
print("你的星座是巨蟹座")
elif (month == 7 and day >= 23) or (month == 8 and day <= 22):
print("你的星座是狮子座")
elif (month == 8 and day >= 23) or (month == 9 and day <= 22):
print("你的星座是处女座")
elif (month == 9 and day >= 23) or (month == 10 and day <= 23):
print("你的星座是天秤座")
elif (month == 10 and day >= 24) or (month == 11 and day <= 22):
print("你的星座是天蝎座")
elif (month == 11 and day >= 23) or (month == 12 and day <= 21):
print("你的星座是射手座")
elif (month == 12 and day >= 22) or (month == 1 and day <= 19):
print("你的星座是摩羯座")
else:
print("输入的日期或月份有误")
```
首先,我们要求用户输入月份和日期,并将其转换为整数类型。然后,使用一系列的if语句来判断输入的月份和日期是否符合某个星座的时间段,如果符合,则输出对应的星座名称。如果不符合,则输出提示信息。
此程序仅适用于西方星座,如果需要包含其他国家或地区的星座,需要根据不同的时间段来进行修改。
阅读全文
相关推荐
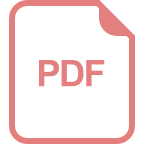

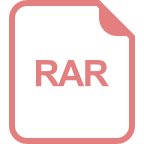
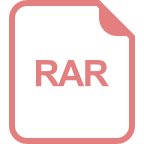
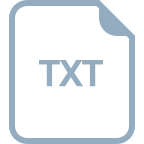
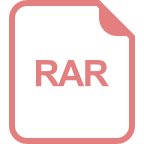
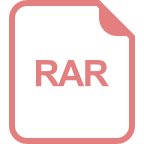
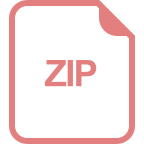







