import numpy as np import tensorflow as tf from tensorflow import keras import matplotlib.pyplot as plt Let us define a plt function for simplicity def plt_loss(x,training_metric,testing_metric,ax,colors = ['b']): ax.plot(x,training_metric,'b',label = 'Train') ax.plot(x,testing_metric,'k',label = 'Test') ax.set_xlabel('Epochs') ax.set_ylabel('Accuracy') plt.legend() plt.grid() plt.show() tf.keras.utils.set_random_seed(1) We import the Minist Dataset using Keras.datasets (train_data, train_labels), (test_data, test_labels) = keras.datasets.mnist.load_data() We first vectorize the image (28*28) into a vector (784) train_data = train_data.reshape(train_data.shape[0],train_data.shape[1]train_data.shape[2]) # 60000784 test_data = test_data.reshape(test_data.shape[0],test_data.shape[1]test_data.shape[2]) # 10000784 We next change label number to a 10 dimensional vector, e.g., 1-> train_labels = keras.utils.to_categorical(train_labels,10) test_labels = keras.utils.to_categorical(test_labels,10) start to build a MLP model N_batch_size = 5000 N_epochs = 100 lr = 0.01 we build a three layer model, 784 -> 64 -> 10 MLP_3 = keras.models.Sequential([ keras.layers.Dense(128, input_shape=(784,),activation='relu'), keras.layers.Dense(64, activation='relu'), keras.layers.Dense(10,activation='softmax') ]) MLP_3.compile( optimizer=keras.optimizers.Adam(lr), loss= 'categorical_crossentropy', metrics = ['accuracy'] ) History = MLP_3.fit(train_data,train_labels, batch_size = N_batch_size, epochs = N_epochs,validation_data=(test_data,test_labels), shuffle=False) train_acc = History.history['accuracy'] test_acc = History.history对于该模型,使用不同数量的训练数据(5000,10000,15000,…,60000,公差=5000的等差数列),绘制训练集和测试集准确率(纵轴)关于训练数据大小(横轴)的曲线
时间: 2024-02-24 09:59:42 浏览: 32
Sure, here's the code:
```
import numpy as np
import tensorflow as tf
from tensorflow import keras
import matplotlib.pyplot as plt
# Define a plt function for simplicity
def plt_loss(x,training_metric,testing_metric,ax,colors = ['b']):
ax.plot(x,training_metric,'b',label = 'Train')
ax.plot(x,testing_metric,'k',label = 'Test')
ax.set_xlabel('Epochs')
ax.set_ylabel('Accuracy')
plt.legend()
plt.grid()
plt.show()
tf.keras.utils.set_random_seed(1)
# Import the MNIST Dataset using Keras.datasets
(train_data, train_labels), (test_data, test_labels) = keras.datasets.mnist.load_data()
# Vectorize the image (28*28) into a vector (784)
train_data = train_data.reshape(train_data.shape[0],train_data.shape[1]*train_data.shape[2]) # 60000*784
test_data = test_data.reshape(test_data.shape[0],test_data.shape[1]*test_data.shape[2]) # 10000*784
# Change label number to a 10 dimensional vector, e.g., 1-> [0,1,0,0,0,0,0,0,0,0]
train_labels = keras.utils.to_categorical(train_labels,10)
test_labels = keras.utils.to_categorical(test_labels,10)
# Start to build a MLP model
N_batch_size = 5000
N_epochs = 100
lr = 0.01
# Build a three layer model, 784 -> 64 -> 10
MLP_3 = keras.models.Sequential([
keras.layers.Dense(128, input_shape=(784,),activation='relu'),
keras.layers.Dense(64, activation='relu'),
keras.layers.Dense(10,activation='softmax')
])
MLP_3.compile(
optimizer=keras.optimizers.Adam(lr),
loss= 'categorical_crossentropy',
metrics = ['accuracy']
)
# Store the training history
train_acc = []
test_acc = []
for i in range(5000,65000,5000):
print('Training on',i,'samples')
History = MLP_3.fit(train_data[:i],train_labels[:i], batch_size = N_batch_size, epochs = N_epochs,validation_data=(test_data,test_labels), shuffle=False)
train_acc.append(History.history['accuracy'][-1])
test_acc.append(History.history['val_accuracy'][-1])
# Plot the training and testing accuracy as a function of training data size
plt.figure(figsize=(8,5))
plt.plot(range(5000,65000,5000),train_acc,'b',label = 'Train')
plt.plot(range(5000,65000,5000),test_acc,'k',label = 'Test')
plt.xlabel('Training Data Size')
plt.ylabel('Accuracy')
plt.legend()
plt.grid()
plt.show()
```
This code trains a three-layer MLP model on the MNIST dataset using Keras. It then loops through different numbers of training samples (5000, 10000, 15000, ..., 60000) and trains the model on each subset of the data for 100 epochs. After each training run, it records the final training and testing accuracy. Finally, it plots a graph of accuracy as a function of training data size.
相关推荐
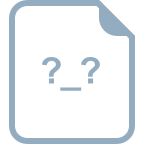
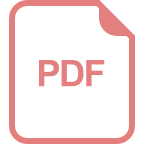
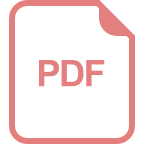















