用DocumentBuilder在word文档末尾按照已有的二级标题顺序添加二级标题
时间: 2024-09-06 19:05:15 浏览: 31
在Java中,可以使用Apache POI库中的`DocumentBuilder`类来操作Word文档(.docx格式)。`DocumentBuilder`提供了一套API来构建和修改Word文档的内容,包括添加标题、段落等。按照你所要求的,在已有的Word文档末尾添加一个二级标题,并保持标题的顺序,可以通过以下步骤实现:
1. 首先,确保你已经将Apache POI的依赖库添加到你的项目中。
2. 使用`XWPFDocument`类加载已有的Word文档。
3. 创建一个`XWPFParagraph`对象,代表新的二级标题。
4. 通过`XWPFParagraph`的样式设置,将其设置为二级标题的样式。
5. 使用`DocumentBuilder`将新创建的二级标题添加到文档的末尾。
下面是一个简单的代码示例:
```java
import org.apache.poi.xwpf.usermodel.*;
import org.apache.poi.openxml4j.exceptions.InvalidFormatException;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class AppendHeading {
public static void main(String[] args) throws IOException, InvalidFormatException {
// 打开现有文档
FileInputStream fis = new FileInputStream("path/to/your/document.docx");
XWPFDocument document = new XWPFDocument(fis);
// 创建一个标题段落
XWPFParagraph newHeading = document.createParagraph();
// 设置标题级别为2
XWPFRun run = newHeading.createRun();
run.setText("新二级标题文本");
newHeading.setStyle("Heading2");
// 将新的标题段落添加到文档末尾
document.createParagraph(); // 添加空段落以避免标题紧跟在文档末尾
// 保存文档
FileOutputStream out = new FileOutputStream("path/to/your/document.docx");
document.write(out);
out.close();
document.close();
fis.close();
}
}
```
请确保将`"path/to/your/document.docx"`替换为你的Word文档的实际路径,并根据需要调整`"新二级标题文本"`的内容。
相关推荐
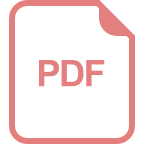
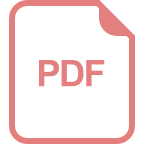
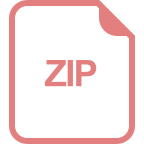














