linux使用父进程创建四个子进程,在进程间用pipe函数进行通信代码怎么写
时间: 2024-12-06 20:21:55 浏览: 8
在Linux中,你可以使用`fork()`系统调用创建子进程,并利用`pipe()`创建管道来实现在进程间的数据通信。下面是一个简单的示例,展示了如何在一个父进程中创建4个子进程,每个子进程读取从父进程通过管道传递过来的信息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <fcntl.h>
#define BUFFER_SIZE 64
void write_to_child(int pipefd[2], char *msg) {
if (write(pipefd[1], msg, strlen(msg) + 1) == -1) {
perror("Error writing to child");
exit(EXIT_FAILURE);
}
}
void read_from_parent(int pipefd[2]) {
char buffer[BUFFER_SIZE];
ssize_t bytes_read;
while ((bytes_read = read(pipefd[0], buffer, BUFFER_SIZE)) > 0) {
printf("Received from parent: %s\n", buffer);
}
if (bytes_read == -1 && !errno) { // EOF reached
printf("Parent closed the connection.\n");
} else if (errno != EAGAIN && errno != EWOULDBLOCK) {
perror("Error reading from parent");
exit(EXIT_FAILURE);
}
}
int main() {
int parent_pipefd[2]; // 父亲的管道句柄
pid_t child_pids[4];
if (pipe(parent_pipefd) == -1) {
perror("Failed to create pipe");
exit(EXIT_FAILURE);
}
for (int i = 0; i < 4; i++) {
child_pids[i] = fork(); // 创建子进程
if (child_pids[i] == -1) {
perror("Fork failed");
exit(EXIT_FAILURE);
}
if (child_pids[i] == 0) { // 子进程
close(parent_pipefd[0]); // 关闭父进程的读端
char message[] = "This is a message from child process " + itoa(i+1); // 每个子进程传递不同的消息
write_to_child(parent_pipefd, message);
exit(EXIT_SUCCESS); // 子进程结束
} else { // 父进程
close(parent_pipefd[1]); // 关闭父进程的写端
wait(NULL); // 等待子进程完成
read_from_parent(parent_pipefd); // 接收所有子进程的消息
}
}
return 0;
}
```
在这个例子中,父进程创建管道,然后分别fork出4个子进程。每个子进程会从管道的读端接收信息并打印出来,而父进程则等待所有的子进程结束后关闭管道,再从写端接收所有子进程发来的消息。
阅读全文
相关推荐
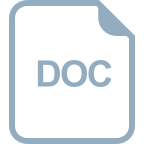
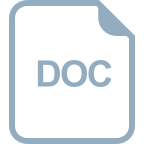
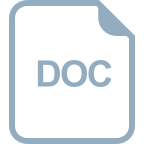





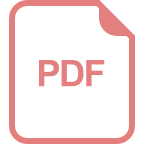
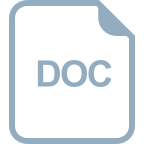
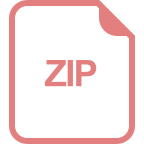
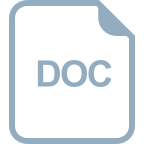
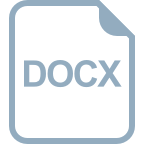
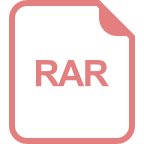
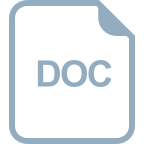
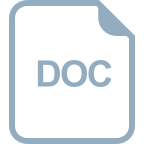
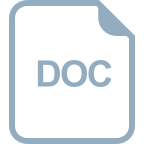

