编写一个程序使用系统调用fork生成3个子进程,并使用系统调用pipe创建一个管道,使得这3个子进程和父进程公用同一管道进行信息通信
时间: 2024-09-10 10:23:45 浏览: 88
在编写这样的程序时,我们将首先使用`fork()`函数创建三个子进程,然后在每个子进程中使用`pipe()`函数创建管道。下面是一个简单的C语言示例,这个程序将在Linux环境下运行:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#define PIPE_SIZE 512
int main() {
pid_t pid[3]; // 存储子进程ID数组
int parent_pipe[2], child_pipe[2][3]; // 父亲和孩子间的两个管道
if (pipe(parent_pipe) == -1) { // 创建父管道
perror("Pipe creation failed");
return 1;
}
for (int i = 0; i < 3; i++) { // fork三次
if ((pid[i] = fork()) == 0) { // 进入子进程
close(parent_pipe[0]); // 关闭读端
if (pipe(child_pipe[i]) == -1) { // 创建子管道
perror("Child pipe creation failed");
exit(1);
}
dup2(child_pipe[i][1], STDOUT_FILENO); // 将写端连接到stdout
write(child_pipe[i][0], "Hello from Child ", strlen("Hello from Child "));
close(child_pipe[i][1]); // 关闭写端
_exit(0); // 退出子进程
} else if (pid[i] > 0) { // 父进程
close(parent_pipe[1]); // 关闭写端
read(parent_pipe[0], "From Parent: ", sizeof("From Parent: ")); // 父亲向管道写入
printf("Received: %s\n", "From Parent: ");
wait(NULL); // 等待子进程结束
} else {
perror("Fork failed");
return 1;
}
}
return 0;
}
```
在这个程序中:
1. 父进程创建了一个管道,并将其读端复制给所有子进程。
2. 每个子进程接收到管道的写端,当它们运行时,会向管道发送一条消息"Hello from Child"。
3. 父进程从管道读取内容,显示接收到的消息"From Parent: "。
阅读全文
相关推荐
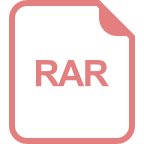
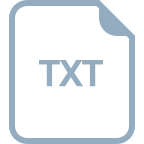
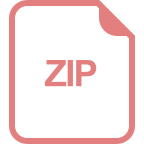















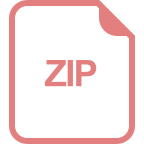