编写一个程序,使用系统调用fork生成3个子进程,并使用系统调用pipe创建一条管道,使得这3个子进程和父进程共用同一管道进行通信
时间: 2024-09-19 07:02:57 浏览: 94
编写这样一个程序需要使用C语言的`fork()`函数创建子进程,以及`pipe()`函数建立管道。下面是一个简单的示例,展示了如何在Unix/Linux环境中实现这个功能:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
#define BUFFER_SIZE 10
void parent(void) {
int pipefd[2]; // 管道文件描述符数组
pid_t child1, child2, child3;
// 创建管道
if (pipe(pipefd) == -1) {
perror("Pipe creation failed");
return;
}
// 创建第一个子进程
child1 = fork();
if (child1 == 0) { // 子进程
close(pipefd[0]); // 关闭读端
write(pipefd[1], "Hello from Child1", strlen("Hello from Child1"));
printf("Child1 wrote to pipe and exited.\n");
exit(0);
} else if (child1 > 0) { // 父进程
close(pipefd[1]); // 父进程关闭写端
// 等待并处理第一个子进程消息
char buffer[BUFFER_SIZE];
read(pipefd[0], buffer, BUFFER_SIZE);
printf("Parent received from Child1: %s\n", buffer);
// 创建第二个子进程
child2 = fork();
if (child2 == 0) {
close(pipefd[0]);
write(pipefd[1], "Hello from Child2", strlen("Hello from Child2"));
printf("Child2 wrote to pipe and exited.\n");
exit(0);
} else if (child2 > 0) {
// 接收第二个子进程消息
read(pipefd[0], buffer, BUFFER_SIZE);
printf("Parent received from Child2: %s\n", buffer);
// 创建第三个子进程
child3 = fork();
if (child3 == 0) {
close(pipefd[0]);
write(pipefd[1], "Hello from Child3", strlen("Hello from Child3"));
printf("Child3 wrote to pipe and exited.\n");
exit(0);
} else if (child3 > 0) {
// 最后一次接收消息
read(pipefd[0], buffer, BUFFER_SIZE);
printf("Parent received from Child3: %s\n", buffer);
}
}
} else {
perror("Forking error");
exit(1);
}
close(pipefd[0]); // 父进程中关闭剩余的管道端口
}
int main() {
parent(); // 调用父进程函数
return 0;
}
```
在这个例子中,父进程首先创建一个管道,然后通过`fork()`函数创建三个子进程。每个子进程会向管道中写入信息,而父进程则从管道读取这些信息。请注意,实际应用中你需要对错误情况进行适当的处理。
阅读全文
相关推荐
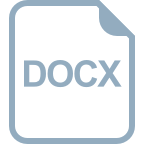
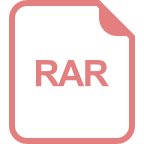
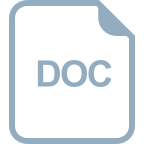












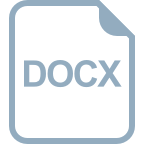