在Linux系统中使用系统调用fork()创建两个子进程,使用系统调用pipe()建立一个管道,两个子进程分别向管道各写一句话: Child process 1 is sending a message! Child process 2 is sending a message! 而父进程则从管道中读出来自于两个子进程的信息,显示在屏幕上。然后分别结束两个子进程的运行。
时间: 2024-03-14 13:43:19 浏览: 17
好的,这是一个关于Linux系统编程的问题。
首先,我们需要使用fork()系统调用创建两个子进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
int main() {
pid_t pid1, pid2;
int pipefd[2];
// 创建管道
if (pipe(pipefd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
// 创建子进程1
pid1 = fork();
if (pid1 == -1) {
perror("fork1");
exit(EXIT_FAILURE);
} else if (pid1 == 0) {
// 子进程1写入信息到管道
close(pipefd[0]); // 关闭管道读端
char *msg = "Child process 1 is sending a message!";
write(pipefd[1], msg, strlen(msg) + 1);
close(pipefd[1]); // 关闭管道写端
exit(EXIT_SUCCESS);
}
// 创建子进程2
pid2 = fork();
if (pid2 == -1) {
perror("fork2");
exit(EXIT_FAILURE);
} else if (pid2 == 0) {
// 子进程2写入信息到管道
close(pipefd[0]); // 关闭管道读端
char *msg = "Child process 2 is sending a message!";
write(pipefd[1], msg, strlen(msg) + 1);
close(pipefd[1]); // 关闭管道写端
exit(EXIT_SUCCESS);
}
// 父进程从管道中读取信息
close(pipefd[1]); // 关闭管道写端
char buf[100];
int num_read;
while ((num_read = read(pipefd[0], buf, 100)) > 0) {
printf("%s\n", buf);
}
close(pipefd[0]); // 关闭管道读端
// 等待子进程结束
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
return 0;
}
```
这段代码首先创建了一个管道,然后使用fork()系统调用创建了两个子进程。子进程1和子进程2分别写入信息到管道中,而父进程则从管道中读取信息并显示在屏幕上。最后,父进程等待两个子进程结束。
注意:为了防止管道被重复读取或写入,需要在使用完管道后关闭管道的读端或写端。同时,为了避免父进程先于子进程结束而造成僵尸进程,需要使用waitpid()等待子进程结束。
相关推荐
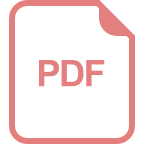
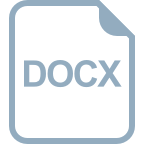
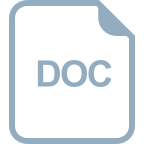














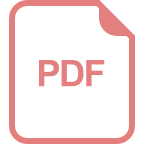