如何在linux系统中实现一个父进程读管道信息,两个字进程写管道信息
时间: 2024-02-15 19:05:47 浏览: 30
在 Linux 系统中,可以使用 `pipe` 函数创建一个管道,然后使用 `fork` 函数创建两个子进程,一个子进程向管道写入数据,另一个子进程也向管道写入数据,父进程则从管道中读取数据。
下面是一个实现的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main(int argc, char *argv[]) {
int fd[2];
pipe(fd); // 创建管道
int pid1 = fork(); // 创建第一个子进程
if (pid1 == 0) { // 子进程1
close(fd[0]); // 关闭管道的读端
char *msg1 = "Hello from child process 1!";
write(fd[1], msg1, strlen(msg1) + 1); // 往管道中写入数据
exit(0);
}
int pid2 = fork(); // 创建第二个子进程
if (pid2 == 0) { // 子进程2
close(fd[0]); // 关闭管道的读端
char *msg2 = "Hello from child process 2!";
write(fd[1], msg2, strlen(msg2) + 1); // 往管道中写入数据
exit(0);
}
close(fd[1]); // 关闭管道的写端
char buf[100];
read(fd[0], buf, sizeof(buf)); // 从管道中读取数据
printf("Received message: %s\n", buf);
read(fd[0], buf, sizeof(buf)); // 从管道中读取数据
printf("Received message: %s\n", buf);
return 0;
}
```
在这个示例代码中,首先通过 `pipe` 函数创建了一个管道,然后使用 `fork` 函数创建了两个子进程。其中,第一个子进程向管道中写入了一条消息,第二个子进程也向管道中写入了一条消息,父进程则从管道中读取了这两条消息,并将其输出到终端上。需要注意的是,子进程向管道中写入数据后,需要调用 `exit` 函数退出,否则它们会继续往下运行,从而会执行到父进程的代码中。
相关推荐
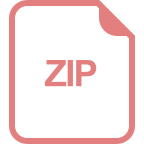















