如何在linux中实现管道的/* 一个父进程写信息,两个子进程读信息 */
时间: 2024-02-15 10:05:14 浏览: 127
在 Linux 中,管道是一种进程间通信机制,可以用于在两个或多个进程之间传输数据。下面是一个示例程序,演示如何在一个父进程中写入信息,并让两个子进程读取信息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main()
{
int fds[2];
pid_t pid1, pid2;
char buf[256];
// 创建管道
if (pipe(fds) == -1) {
perror("pipe");
return 1;
}
// 创建第一个子进程
if ((pid1 = fork()) == -1) {
perror("fork");
return 1;
} else if (pid1 == 0) {
// 子进程1从管道中读取信息
close(fds[1]);
read(fds[0], buf, sizeof(buf));
printf("Child 1 read: %s", buf);
close(fds[0]);
exit(0);
}
// 创建第二个子进程
if ((pid2 = fork()) == -1) {
perror("fork");
return 1;
} else if (pid2 == 0) {
// 子进程2从管道中读取信息
close(fds[1]);
read(fds[0], buf, sizeof(buf));
printf("Child 2 read: %s", buf);
close(fds[0]);
exit(0);
}
// 父进程向管道中写入信息
close(fds[0]);
write(fds[1], "Hello, world!\n", 14);
close(fds[1]);
// 等待子进程退出
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
return 0;
}
```
在上面的程序中,首先创建了一个管道,然后创建了两个子进程。子进程1和子进程2分别从管道中读取信息,并在读取到信息后输出到标准输出。父进程向管道中写入信息,然后等待两个子进程退出。需要注意的是,在读取完信息后,子进程需要关闭管道的读端,否则会一直阻塞在读取操作上。同理,在写入完信息后,父进程需要关闭管道的写端。
阅读全文
相关推荐
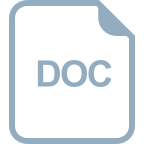
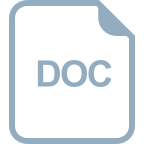
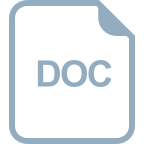

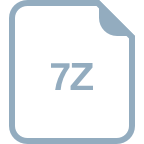













