编写一段程序,实现进程的管道通信。使用系统调用pipe()建立一条管道,2个子进程分别循环向这条管道写一句话:child1 is sending a message! Child2 is sending a message!而父进程则循环从管道中读出信息,显示在屏幕上
时间: 2024-10-18 21:22:21 浏览: 48
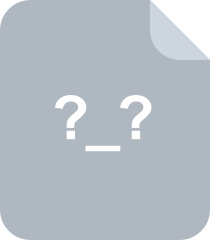
(修改版)进程的管道通信编制一段程序,实现进程的管道通信,使用系统调用pipe()建立一个管道文件;两个子进程P1和P2。

在Unix/Linux环境中,我们可以使用`fork()`、`execve()`、`close()`、`write()`和`read()`等系统调用来实现管道通信。这里是一个简单的Python示例,它展示了如何创建两个子进程通过管道互相发送消息,并由父进程接收并打印出来:
```python
import os
import sys
# 创建管道
def create_pipe():
pipe_fd = os.pipe()
return pipe_fd[0], pipe_fd[1]
# 父进程函数
def parent(child1, child2):
while True:
try:
msg = os.read(child1, 1024)
print(f"Parent received: {msg.decode().strip()}")
except BlockingIOError:
pass
# 子进程1函数
def child1(pipe_r, child2):
os.write(child2, b"Child1 is sending a message!\n")
# 使用os.wait()等待子进程2结束,然后退出
os.waitpid(child2, 0)
os.close(pipe_r)
# 子进程2函数
def child2(pipe_w):
os.close(pipe_w) # 关闭写端
os.write(sys.stdout.fileno(), b"Child2 is sending a message!\n") # 直接写入stdout
os._exit(0) # 立即退出,防止阻塞父进程
if __name__ == "__main__":
# 创建管道
pipe_r, pipe_w = create_pipe()
# fork两次,一次创建子进程1,一次创建子进程2
pid_child1 = os.fork()
if pid_child1 == 0:
child1(pipe_r, -1) # -1 表示关闭父进程的读端
else:
pid_child2 = os.fork()
if pid_child2 == 0:
child2(pipe_w)
else:
parent(pipe_r, -1) # -1 表示关闭子进程1的写端
# 等待所有子进程结束
os.waitpid(pid_child1, 0)
os.waitpid(pid_child2, 0)
```
运行这个程序,你会看到“Parent received: Child1 is sending a message!”和“Parent received: Child2 is sending a message!”交替出现在屏幕上。
阅读全文
相关推荐
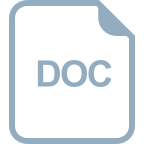
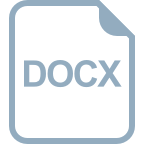
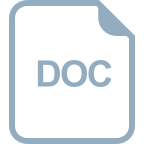
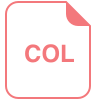
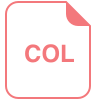
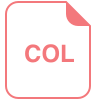
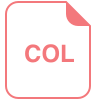
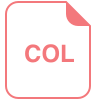
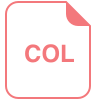
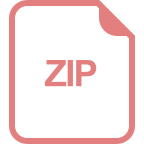
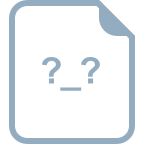
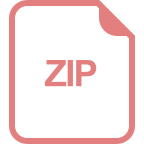
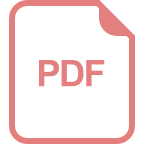
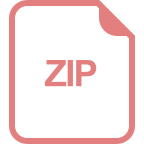
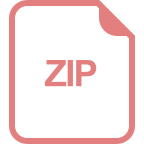
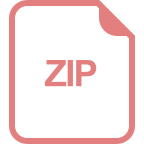