编写一段程序,使用系统调用pipe()建立一条管道线,同时父进程生成2个子进程分别向这条管道写一句话:child1 is sending a message! Child2 is sending a message! 父进程则循环从管道中读出信息,显示在屏幕上。(提示:两个子进程向管道中写入字符的时候要保持互斥,使用lockf)
时间: 2024-02-13 20:06:06 浏览: 160
好的,以下是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
int main()
{
int fd[2];
int ret = pipe(fd); // 创建管道
if (ret < 0) {
perror("pipe error");
exit(1);
}
pid_t pid1, pid2;
pid1 = fork(); // 创建第一个子进程
if (pid1 < 0) {
perror("fork error");
exit(1);
} else if (pid1 == 0) {
// 子进程1
close(fd[0]); // 关闭读端
lockf(fd[1], F_LOCK, 0); // 加锁
write(fd[1], "child1 is sending a message!", 29);
lockf(fd[1], F_ULOCK, 0); // 解锁
close(fd[1]);
exit(0);
} else {
pid2 = fork(); // 创建第二个子进程
if (pid2 < 0) {
perror("fork error");
exit(1);
} else if (pid2 == 0) {
// 子进程2
close(fd[0]); // 关闭读端
lockf(fd[1], F_LOCK, 0); // 加锁
write(fd[1], "child2 is sending a message!", 29);
lockf(fd[1], F_ULOCK, 0); // 解锁
close(fd[1]);
exit(0);
} else {
// 父进程
close(fd[1]); // 关闭写端
char buf[30];
while (1) {
ret = read(fd[0], buf, sizeof(buf)); // 从管道中读取数据
if (ret == 0) {
break;
}
buf[ret] = '\0';
printf("%s\n", buf); // 打印读取到的数据
}
close(fd[0]);
waitpid(pid1, NULL, 0); // 等待子进程1结束
waitpid(pid2, NULL, 0); // 等待子进程2结束
exit(0);
}
}
}
```
以上代码中,我们首先使用 `pipe()` 系统调用创建了一个管道,然后创建了两个子进程,分别向管道中写入一句话,使用 `lockf()` 函数保证了两个子进程对管道的互斥访问。最后,父进程循环从管道中读取数据,并打印输出到屏幕上。注意,我们在父进程中需要等待两个子进程的结束,这里使用 `waitpid()` 函数实现,以免出现僵尸进程。
阅读全文
相关推荐
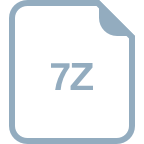
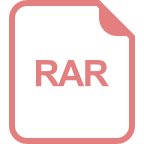
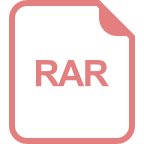
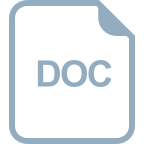
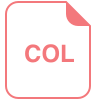
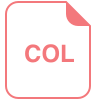
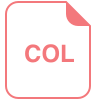
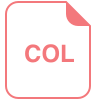
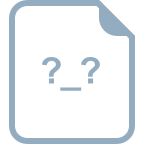
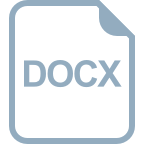
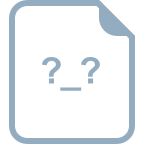
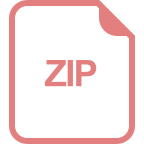
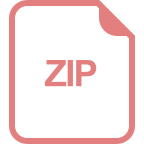
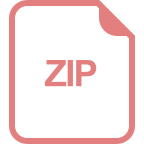
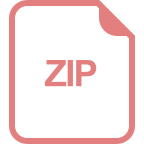
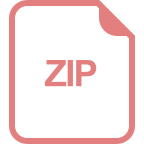