c语言用链表且简洁的实现集合的交并补差运算代码
时间: 2023-06-04 16:04:37 浏览: 90
好的,以下是用单链表实现集合的交并补差运算的代码。代码中使用了结构体表示单链表节点和集合元素:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
typedef struct Set {
Node* head;
int size;
} Set;
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
Set* createSet() {
Set* newSet = (Set*)malloc(sizeof(Set));
newSet->head = NULL;
newSet->size = 0;
return newSet;
}
void addNode(Set* set, int data) {
Node* newNode = createNode(data);
newNode->next = set->head;
set->head = newNode;
set->size++;
}
void printSet(Set* set) {
printf("{ ");
Node* curr = set->head;
while (curr != NULL) {
printf("%d ", curr->data);
curr = curr->next;
}
printf("}\n");
}
Set* intersection(Set* set1, Set* set2) {
Set* newSet = createSet();
Node* curr1 = set1->head;
while (curr1 != NULL) {
Node* curr2 = set2->head;
while (curr2 != NULL) {
if (curr1->data == curr2->data) {
addNode(newSet, curr1->data);
break;
}
curr2 = curr2->next;
}
curr1 = curr1->next;
}
return newSet;
}
Set* unionSet(Set* set1, Set* set2) {
Set* newSet = createSet();
Node* curr1 = set1->head;
while (curr1 != NULL) {
addNode(newSet, curr1->data);
curr1 = curr1->next;
}
Node* curr2 = set2->head;
while (curr2 != NULL) {
Node* currNew = newSet->head;
int exists = 0;
while (currNew != NULL) {
if (curr2->data == currNew->data) {
exists = 1;
break;
}
currNew = currNew->next;
}
if (!exists) {
addNode(newSet, curr2->data);
}
curr2 = curr2->next;
}
return newSet;
}
Set* complement(Set* set1, Set* set2) {
Set* newSet = createSet();
Node* curr1 = set1->head;
while (curr1 != NULL) {
Node* curr2 = set2->head;
int exists = 0;
while (curr2 != NULL) {
if (curr1->data == curr2->data) {
exists = 1;
break;
}
curr2 = curr2->next;
}
if (!exists) {
addNode(newSet, curr1->data);
}
curr1 = curr1->next;
}
return newSet;
}
Set* difference(Set* set1, Set* set2) {
Set* newSet = createSet();
Node* curr1 = set1->head;
while (curr1 != NULL) {
Node* curr2 = set2->head;
int exists = 0;
while (curr2 != NULL) {
if (curr1->data == curr2->data) {
exists = 1;
break;
}
curr2 = curr2->next;
}
if (!exists) {
addNode(newSet, curr1->data);
}
curr1 = curr1->next;
}
curr1 = set2->head;
while (curr1 != NULL) {
Node* curr2 = set1->head;
int exists = 0;
while (curr2 != NULL) {
if (curr1->data == curr2->data) {
exists = 1;
break;
}
curr2 = curr2->next;
}
if (!exists) {
addNode(newSet, curr1->data);
}
curr1 = curr1->next;
}
return newSet;
}
int main() {
Set* set1 = createSet();
Set* set2 = createSet();
addNode(set1, 1);
addNode(set1, 3);
addNode(set1, 5);
addNode(set1, 7);
addNode(set2, 2);
addNode(set2, 4);
addNode(set2, 6);
addNode(set2, 8);
printf("Set1: ");
printSet(set1);
printf("Set2: ");
printSet(set2);
Set* inter = intersection(set1, set2);
printf("Intersection: ");
printSet(inter);
Set* uni = unionSet(set1, set2);
printf("Union: ");
printSet(uni);
Set* com = complement(set1, set2);
printf("Complement: ");
printSet(com);
Set* diff = difference(set1, set2);
printf("Difference: ");
printSet(diff);
return 0;
}
```
相关推荐
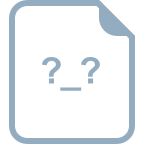
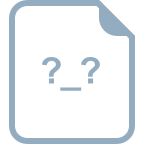
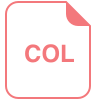
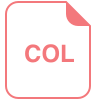
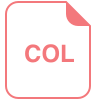
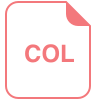
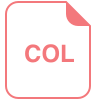









