用Java写一个放烟花的代码
时间: 2024-05-07 10:19:28 浏览: 14
以下是一个简单的 Java 代码,用于放烟花:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Fireworks extends JFrame {
private static final long serialVersionUID = 1L;
private List<Particle> particles = new ArrayList<Particle>();
private Random random = new Random();
private int counter = 0;
public Fireworks() {
setTitle("Fireworks");
setSize(800, 600);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel() {
private static final long serialVersionUID = 1L;
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
for (Particle particle : particles) {
particle.draw(g);
}
}
};
setContentPane(panel);
setVisible(true);
run();
}
private void run() {
while (true) {
if (counter++ % 10 == 0) {
if (random.nextInt(100) < 30) {
particles.add(new Firework(random.nextInt(getWidth()), getHeight() - 1));
}
}
for (Particle particle : particles) {
particle.move();
}
particles.removeIf(p -> p.isDead());
repaint();
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
private interface Particle {
void move();
void draw(Graphics g);
boolean isDead();
}
private class Firework implements Particle {
private int x;
private int y;
private int age;
private int maxAge;
private List<Spark> sparks = new ArrayList<Spark>();
public Firework(int x, int y) {
this.x = x;
this.y = y;
maxAge = random.nextInt(60) + 60;
}
@Override
public void move() {
if (age++ < maxAge) {
y--;
} else {
for (int i = 0; i < 20; i++) {
sparks.add(new Spark(x, y));
}
}
for (Spark spark : sparks) {
spark.move();
}
}
@Override
public void draw(Graphics g) {
if (age < maxAge) {
g.setColor(Color.WHITE);
g.drawLine(x, y, x, y);
} else {
for (Spark spark : sparks) {
spark.draw(g);
}
}
}
@Override
public boolean isDead() {
return age > maxAge && sparks.isEmpty();
}
}
private class Spark implements Particle {
private Point position;
private Point velocity;
private int age;
private int maxAge;
private Color color;
public Spark(int x, int y) {
position = new Point(x, y);
velocity = new Point(random.nextInt(5) - 2, random.nextInt(5) - 8);
maxAge = random.nextInt(20) + 20;
color = new Color(random.nextInt(255), random.nextInt(255), random.nextInt(255));
}
@Override
public void move() {
position.translate(velocity.x, velocity.y);
velocity.translate(0, 1);
age++;
}
@Override
public void draw(Graphics g) {
g.setColor(color);
g.drawLine(position.x, position.y, position.x, position.y);
}
@Override
public boolean isDead() {
return age > maxAge;
}
}
public static void main(String[] args) {
new Fireworks();
}
}
```
这个程序使用 Java Swing 绘制烟花。它创建了一个 `Fireworks` 类来启动程序,该类继承自 `JFrame`,并在其中创建一个 `JPanel` 作为内容面板。这个程序在一个无限循环中运行,每隔一段时间就会发射一个烟花。烟花会发射出许多火花,火花会向上飞,然后开始下落,最终消失。
相关推荐









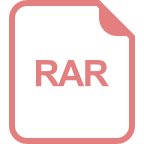
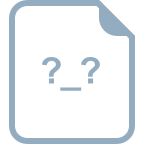
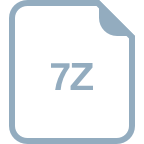
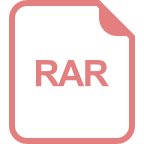
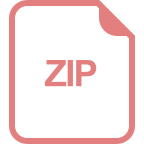