用java写一个烟花绽放的代码
时间: 2023-03-24 11:01:29 浏览: 282
以下是使用 Java 编写的烟花绽放的代码示例:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.util.ArrayList;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Fireworks extends JPanel implements Runnable {
private ArrayList<Particle> particles = new ArrayList<Particle>();
private Point explosionPos;
private boolean exploded = false;
public Fireworks() {
setBackground(Color.BLACK);
setDoubleBuffered(true);
}
public void run() {
try {
Thread.sleep(3000); // Wait for 3 seconds before exploding
} catch (InterruptedException e) {
e.printStackTrace();
}
explode();
while (true) {
update();
repaint();
try {
Thread.sleep(15);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
private void explode() {
explosionPos = new Point(getWidth() / 2, getHeight() / 2);
for (int i = 0; i < 200; i++) {
particles.add(new Particle(explosionPos));
}
exploded = true;
}
private void update() {
if (exploded) {
for (int i = particles.size() - 1; i >= 0; i--) {
Particle p = particles.get(i);
if (p.shouldRemove()) {
particles.remove(i);
} else {
p.update();
}
}
if (particles.size() == 0) {
exploded = false;
}
}
}
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(Color.WHITE);
for (Particle p : particles) {
p.draw(g);
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Fireworks");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(500, 500);
Fireworks fireworks = new Fireworks();
frame.add(fireworks);
frame.setVisible(true);
new Thread(fireworks).start();
}
private class Particle {
private Point pos;
private int size;
private Color color;
private int dx, dy;
private int life;
public Particle(Point pos) {
this.pos = new Point(pos);
size = (int) (Math.random() * 5) + 5;
color = new Color((int) (Math.random() * 256), (int) (Math.random() * 256), (int) (Math.random() * 256));
dx = (int) (Math.random() * 5) - 2;
dy = (int) (Math.random() * 5) - 2;
life = (int) (Math.random() * 30) + 30;
}
public void update() {
pos.x += dx;
pos.y += dy;
life--;
}
public boolean shouldRemove() {
return life <= 0;
}
public void draw(Graphics g) {
g.setColor(color);
g.fillOval(pos.x, pos.y, size, size);
}
}
}
```
这个程序实现了一个简单的烟花效果,包括烟花绽放和粒子的运动。在程序运行时,它将等待 3 秒钟,然后烟花将绽放并开始运动。运行过程中,粒子会逐渐消失
相关推荐
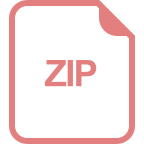
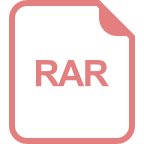
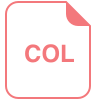
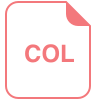












