用前端代码写一个告白气球动画的代码
时间: 2023-09-12 21:05:06 浏览: 210
### 回答1:
好的,我可以回答这个问题。以下是一个简单的告白气球动画的前端代码:
HTML 代码:
```
<div class="balloon-container">
<div class="balloon"></div>
<div class="string"></div>
</div>
```
CSS 代码:
```
.balloon-container {
position: relative;
width: 200px;
height: 300px;
}
.balloon {
position: absolute;
top: ;
left: 50%;
transform: translateX(-50%);
width: 100px;
height: 150px;
border-radius: 50% 50% ;
background-color: pink;
}
.string {
position: absolute;
top: 150px;
left: 50%;
transform: translateX(-50%);
width: 2px;
height: 150px;
background-color: black;
}
```
JavaScript 代码:
```
const balloon = document.querySelector('.balloon');
const string = document.querySelector('.string');
balloon.addEventListener('click', () => {
balloon.classList.add('float');
string.classList.add('swing');
});
```
这段代码会在点击气球时,给气球和气球的线条添加动画效果,让气球漂浮并摇摆。
### 回答2:
以下是用前端代码实现一个简单的告白气球动画的示例代码。在这个示例中,我使用了HTML、CSS和JavaScript来创建动画效果。
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>告白气球动画</title>
<style>
#balloon {
position: absolute;
top: 100px;
left: 50%;
transform: translateX(-50%);
width: 100px;
height: 200px;
background-color: red;
border-radius: 50%;
transition: top 5s;
}
@keyframes float {
0% {
top: 100px;
}
50% {
top: 50px;
}
100% {
top: 100px;
}
}
</style>
</head>
<body>
<div id="balloon"></div>
<script>
const balloon = document.getElementById('balloon');
balloon.addEventListener('click', () => {
balloon.style.animation = 'float 5s ease-in-out infinite';
});
</script>
</body>
</html>
```
CSS部分的代码用于设置气球的样式,使其成为一个圆形红色元素,并添加了一个动画过渡属性来实现气球上下浮动的效果。
JavaScript部分代码则为气球添加了一个点击事件监听器,当点击气球时,会为气球添加一个浮动动画效果。点击后的气球将不断上下浮动,直到重新点击气球或刷新页面。
以上代码可以在浏览器中运行,并且可以随时点击气球启动动画效果。当点击气球时,气球将会在垂直方向上一直上下浮动,给人一种如同漂浮的气球的效果。这是一个简单的告白气球动画示例,你可以根据自己的需求进行修改和扩展。
### 回答3:
如下是一个用前端代码实现告白气球动画的示例:
```html
<!DOCTYPE html>
<html>
<head>
<style>
#balloon {
position: absolute;
top: 200px;
left: 0px;
transition: left 5s linear;
}
</style>
</head>
<body>
<div id="balloon">
<img src="balloon.png" alt="Balloon" width="100px" height="150px">
</div>
<script>
const balloon = document.getElementById("balloon");
const windowWidth = window.innerWidth;
function animateBalloon() {
balloon.style.left = (windowWidth - 100) + "px"; // 将气球移到屏幕最右侧
setTimeout(function() {
balloon.style.top = "50px"; // 将气球上升
}, 5000);
setTimeout(function() {
balloon.style.top = "200px"; // 气球重新下降
balloon.style.left = "0px"; // 气球返回原位
}, 10000);
}
animateBalloon();
</script>
</body>
</html>
```
上述代码使用HTML和CSS来创建一个页面,并使用JavaScript来实现气球的动画效果。在页面上,我们有一个带有id "balloon" 的div元素,其中包含一个气球的图像。通过将气球的位置定位为绝对值,我们可以通过调整其"left"和"top"属性来控制其移动。通过CSS中的过渡属性,我们可以实现平滑的移动效果。
在JavaScript中,我们首先获取到气球的div元素,并获取到当前浏览器窗口的宽度。然后,通过更改气球的"left"属性,我们将气球移动到屏幕最右侧。接下来,我们使用setTimeout函数,在5秒钟后将气球的"top"属性更改为一个较小的值,以实现气球的上升效果。最后,在10秒后,我们将气球的"top"和"left"属性更改为原始值,以使气球返回原位。
通过执行animateBalloon函数,我们可以启动气球动画。
阅读全文
相关推荐
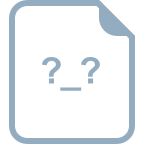
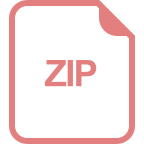
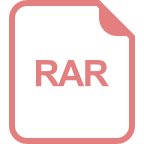
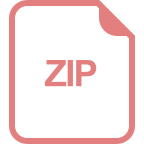
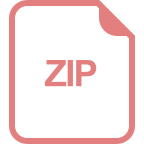
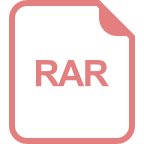
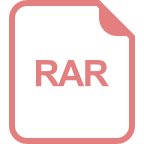
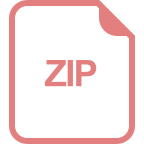
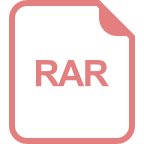
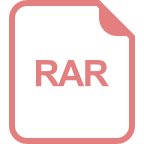
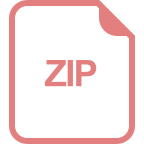
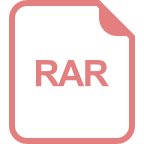
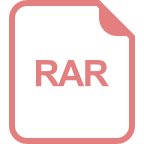
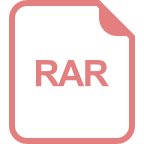
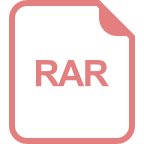
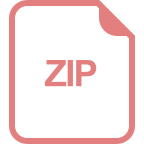
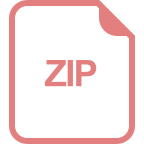
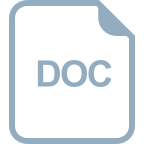