processing十个运用三角函数制作的案例
时间: 2023-10-27 19:04:39 浏览: 104
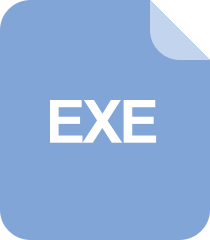
三角函数图例程序

下面是十个使用三角函数制作的案例:
1. 绘制正弦函数的波形
```
float angle = 0;
float angle_increment = 0.1;
void setup() {
size(400, 400);
}
void draw() {
background(255);
stroke(0);
noFill();
beginShape();
for (int i = 0; i < width; i++) {
float y = sin(angle) * 100 + height/2;
vertex(i, y);
angle += angle_increment;
}
endShape();
}
```
2. 制作一个旋转的正方形
```
float angle = 0;
float angle_increment = 0.1;
void setup() {
size(400, 400);
}
void draw() {
background(255);
translate(width/2, height/2);
rotate(angle);
rectMode(CENTER);
rect(0, 0, 100, 100);
angle += angle_increment;
}
```
3. 制作一个辐射状的图形
```
void setup() {
size(400, 400);
}
void draw() {
background(255);
translate(width/2, height/2);
for (int i = 0; i < 12; i++) {
rotate(TWO_PI/12);
line(0, 0, 0, 100);
}
}
```
4. 制作一个动态的星形
```
float angle = 0;
float angle_increment = 0.1;
void setup() {
size(400, 400);
}
void draw() {
background(255);
translate(width/2, height/2);
beginShape();
for (int i = 0; i < 5; i++) {
float x = sin(angle + TWO_PI/5*i) * 100;
float y = cos(angle + TWO_PI/5*i) * 100;
vertex(x, y);
}
endShape(CLOSE);
angle += angle_increment;
}
```
5. 制作一个波浪形的图形
```
float angle = 0;
float angle_increment = 0.1;
float amplitude = 50;
void setup() {
size(400, 400);
}
void draw() {
background(255);
translate(0, height/2);
noFill();
beginShape();
for (int i = 0; i < width; i++) {
float y = sin(angle + TWO_PI*i/width) * amplitude;
vertex(i, y);
}
endShape();
angle += angle_increment;
}
```
6. 制作一个旋转的圆形
```
float angle = 0;
float angle_increment = 0.1;
void setup() {
size(400, 400);
}
void draw() {
background(255);
translate(width/2, height/2);
rotate(angle);
ellipse(0, 0, 100, 100);
angle += angle_increment;
}
```
7. 制作一个三角形的旋转动画
```
float angle = 0;
float angle_increment = 0.1;
void setup() {
size(400, 400);
}
void draw() {
background(255);
translate(width/2, height/2);
rotate(angle);
triangle(-50, -50, 50, -50, 0, 50);
angle += angle_increment;
}
```
8. 制作一个旋转的星形
```
float angle = 0;
float angle_increment = 0.1;
void setup() {
size(400, 400);
}
void draw() {
background(255);
translate(width/2, height/2);
rotate(angle);
beginShape();
for (int i = 0; i < 5; i++) {
float x = sin(TWO_PI/5*i) * 100;
float y = cos(TWO_PI/5*i) * 100;
vertex(x, y);
}
endShape(CLOSE);
angle += angle_increment;
}
```
9. 制作一个旋转的花瓣图案
```
float angle = 0;
float angle_increment = 0.1;
void setup() {
size(400, 400);
}
void draw() {
background(255);
translate(width/2, height/2);
rotate(angle);
beginShape();
for (int i = 0; i < 6; i++) {
float x = sin(TWO_PI/6*i) * 100;
float y = cos(TWO_PI/6*i) * 100;
vertex(x, y);
vertex(x*0.5, y*0.5);
}
endShape(CLOSE);
angle += angle_increment;
}
```
10. 制作一个动态的螺旋线
```
float angle = 0;
float angle_increment = 0.1;
float radius = 0;
float radius_increment = 1;
void setup() {
size(400, 400);
}
void draw() {
background(255);
translate(width/2, height/2);
float x = cos(angle) * radius;
float y = sin(angle) * radius;
ellipse(x, y, 10, 10);
angle += angle_increment;
radius += radius_increment;
}
```
阅读全文
相关推荐
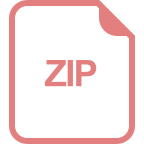
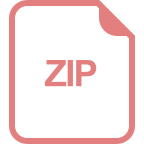






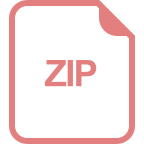
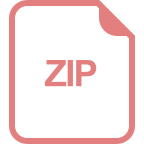
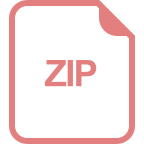

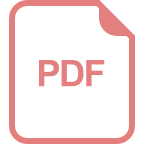
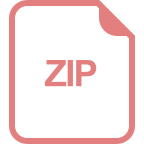
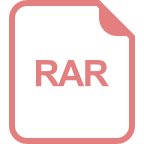
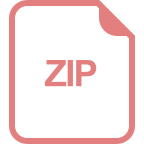