processing十个运用三角函数的动画
时间: 2023-11-25 21:07:55 浏览: 142
以下是一个使用三角函数创建动画的 Processing 代码示例:
```java
float angle = 0;
void setup() {
size(400, 400);
smooth();
}
void draw() {
background(255);
// 计算 x 和 y 的值
float x = cos(angle) * 100 + width/2;
float y = sin(angle) * 100 + height/2;
// 绘制圆形
ellipse(x, y, 50, 50);
// 增加角度
angle += 0.1;
}
```
此代码将在屏幕中央绘制一个随时间变化的圆形。可以通过调整 `angle` 的增量来控制动画速度,也可以通过调整圆形的半径和位置来创建不同的动画效果。
阅读全文
相关推荐
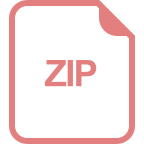
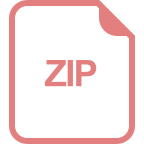
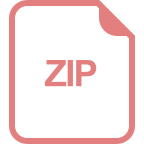
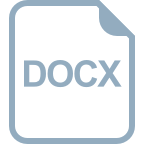
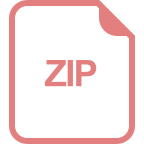
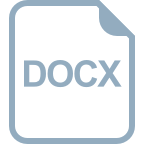
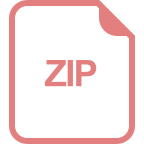
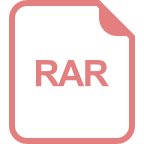
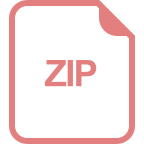
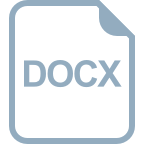
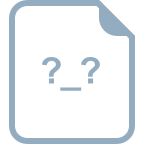
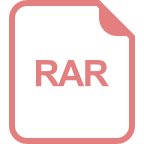
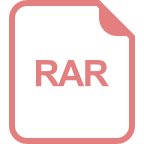
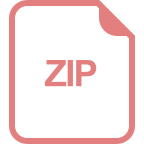
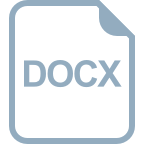