processing十个运用三角函数的案例
时间: 2023-10-27 14:04:39 浏览: 152
以下是十个使用三角函数的 Processing 案例:
1. 通过正弦函数创建一个波浪动画
```processing
float angle = 0;
float[] yvalues;
void setup() {
size(640, 360);
yvalues = new float[width];
}
void draw() {
background(0);
calcWave();
renderWave();
}
void calcWave() {
angle += 0.05;
float x = angle;
for (int i = 0; i < yvalues.length; i++) {
yvalues[i] = sin(x) * 100;
x += 0.1;
}
}
void renderWave() {
noStroke();
fill(255);
for (int x = 0; x < yvalues.length; x++) {
ellipse(x, height / 2 + yvalues[x], 16, 16);
}
}
```
2. 使用正弦和余弦函数创建一个旋转的球
```processing
float angle = 0;
void setup() {
size(640, 360, P3D);
}
void draw() {
background(0);
translate(width / 2, height / 2, 0);
rotateX(angle);
rotateY(angle * 1.3);
fill(255, 0, 150);
sphere(100);
angle += 0.03;
}
```
3. 使用正弦函数创建一个彩虹效果的线条
```processing
float xspacing = 8;
float w;
float amplitude = 75.0;
float period = 150.0;
float[] theta;
float[] yvalues;
void setup() {
size(640, 360);
w = width + 16;
theta = new float[int(w / xspacing)];
yvalues = new float[int(w / xspacing)];
}
void draw() {
background(0);
calcWave();
renderWave();
}
void calcWave() {
float dx = (TWO_PI / period) * xspacing;
for (int i = 0; i < theta.length; i++) {
theta[i] += dx;
yvalues[i] = sin(theta[i]) * amplitude;
}
}
void renderWave() {
noFill();
stroke(255);
strokeWeight(2);
beginShape();
for (int x = 0; x < yvalues.length; x++) {
vertex(x * xspacing, height / 2 + yvalues[x]);
}
endShape();
}
```
4. 使用正切函数创建一个螺旋线
```processing
float angle = 0;
float scalar = 10;
void setup() {
size(640, 360);
}
void draw() {
background(0);
translate(width / 2, height / 2);
for (int i = 0; i < 20; i++) {
float radius = i * scalar;
float x = radius * cos(angle * i / 10);
float y = radius * sin(angle * i / 10);
noStroke();
fill(i * 10, 0, 255 - i * 10);
ellipse(x, y, i, i);
}
angle += 0.05;
}
```
5. 使用余弦函数和正弦函数创建一个心形图案
```processing
float angle = 0;
float scalar = 100;
void setup() {
size(640, 360);
background(255);
noStroke();
fill(255, 0, 0);
}
void draw() {
float x = scalar * pow(sin(angle), 3);
float y = -scalar * (cos(angle) - 1) * sin(angle);
ellipse(x + width / 2, y + height / 2, 16, 16);
angle += 0.05;
}
```
6. 使用正切函数创建一个机械臂的动画
```processing
float angle1 = 0;
float angle2 = 0;
void setup() {
size(640, 360);
}
void draw() {
background(0);
float x1 = 100 * cos(angle1);
float y1 = 100 * sin(angle1);
float x2 = x1 + 100 * cos(angle1 + angle2);
float y2 = y1 + 100 * sin(angle1 + angle2);
stroke(255);
strokeWeight(4);
line(0, 0, x1, y1);
line(x1, y1, x2, y2);
noStroke();
fill(255);
ellipse(x1, y1, 16, 16);
ellipse(x2, y2, 16, 16);
angle1 += 0.05;
angle2 += 0.1;
}
```
7. 使用正切函数创建一个纸飞机的动画
```processing
float angle = 0;
void setup() {
size(640, 360);
}
void draw() {
background(0);
translate(width / 2, height / 2);
rotate(angle);
fill(255);
triangle(-30, 0, 30, 0, 0, -60);
angle += atan2(mouseY - height / 2, mouseX - width / 2) - angle;
}
```
8. 使用正弦函数创建一个音乐可视化效果
```processing
import ddf.minim.*;
Minim minim;
AudioPlayer song;
float[] fft;
void setup() {
size(640, 360);
minim = new Minim(this);
song = minim.loadFile("song.mp3");
song.play();
fft = new float[song.bufferSize() / 2];
}
void draw() {
background(0);
stroke(255);
noFill();
strokeWeight(2);
song.getFFT(fft);
beginShape();
for (int i = 0; i < fft.length; i++) {
float x = map(i, 0, fft.length, 0, width);
float y = map(fft[i], 0, 255, height, 0);
curveVertex(x, y + sin(x * 0.01) * 50);
}
endShape();
}
```
9. 使用余弦函数和正弦函数创建一个星星的动画
```processing
float angle = 0;
void setup() {
size(640, 360);
}
void draw() {
background(0);
translate(width / 2, height / 2);
for (int i = 0; i < 5; i++) {
float x = 100 * cos(angle + i * TWO_PI / 5);
float y = 100 * sin(angle + i * TWO_PI / 5);
noStroke();
fill(255);
ellipse(x, y, 16, 16);
stroke(255);
strokeWeight(2);
line(0, 0, x, y);
}
angle += 0.1;
}
```
10. 使用反正弦函数创建一个拖动的球体
```processing
float x = 0;
float y = 0;
float r = 50;
float angle = 0;
void setup() {
size(640, 360);
}
void draw() {
background(0);
x = r * cos(angle);
y = r * sin(angle);
noStroke();
fill(255);
ellipse(width / 2 + x, height / 2 + y, 32, 32);
angle = asin((mouseY - height / 2) / r);
if (mouseX < width / 2) {
angle = PI - angle;
}
}
```
阅读全文
相关推荐
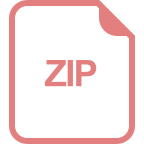
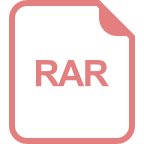
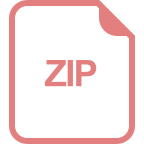








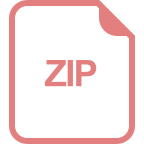
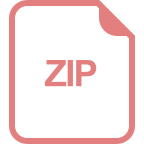
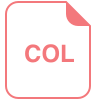
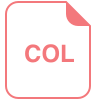
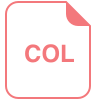
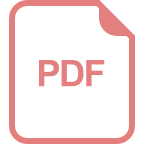
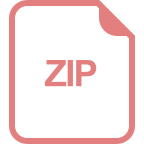