processing十个运用三角函数的动画案例
时间: 2023-11-25 18:07:55 浏览: 69
以下是十个使用三角函数创建动画的 Processing 代码示例:
1. 绘制旋转的正方形
```java
float angle = 0;
void setup() {
size(400, 400);
smooth();
}
void draw() {
background(255);
// 计算正方形的四个顶点的位置
float x1 = cos(angle) * 100 + width/2;
float y1 = sin(angle) * 100 + height/2;
float x2 = cos(angle + PI/2) * 100 + width/2;
float y2 = sin(angle + PI/2) * 100 + height/2;
float x3 = cos(angle + PI) * 100 + width/2;
float y3 = sin(angle + PI) * 100 + height/2;
float x4 = cos(angle + 3*PI/2) * 100 + width/2;
float y4 = sin(angle + 3*PI/2) * 100 + height/2;
// 绘制正方形
quad(x1, y1, x2, y2, x3, y3, x4, y4);
// 增加角度
angle += 0.05;
}
```
2. 绘制螺旋线
```java
float angle = 0;
void setup() {
size(400, 400);
smooth();
}
void draw() {
background(255);
// 计算螺旋线的坐标
float x = cos(angle) * angle * 2 + width/2;
float y = sin(angle) * angle * 2 + height/2;
// 绘制圆形
ellipse(x, y, 5, 5);
// 增加角度
angle += 0.05;
}
```
3. 绘制心形线
```java
float angle = 0;
void setup() {
size(400, 400);
smooth();
}
void draw() {
background(255);
// 计算心形线的坐标
float x = 16 * pow(sin(angle), 3);
float y = -13 * cos(angle) + 5 * cos(2 * angle) + 2 * cos(3 * angle) + cos(4 * angle);
x = x * 20 + width/2;
y = y * 20 + height/2;
// 绘制圆形
ellipse(x, y, 5, 5);
// 增加角度
angle += 0.05;
}
```
4. 绘制星形
```java
float angle = 0;
void setup() {
size(400, 400);
smooth();
}
void draw() {
background(255);
// 计算星形的坐标
float x1 = cos(angle) * 100 + width/2;
float y1 = sin(angle) * 100 + height/2;
float x2 = cos(angle + 2*PI/5) * 100 + width/2;
float y2 = sin(angle + 2*PI/5) * 100 + height/2;
float x3 = cos(angle + 4*PI/5) * 100 + width/2;
float y3 = sin(angle + 4*PI/5) * 100 + height/2;
float x4 = cos(angle + 6*PI/5) * 100 + width/2;
float y4 = sin(angle + 6*PI/5) * 100 + height/2;
float x5 = cos(angle + 8*PI/5) * 100 + width/2;
float y5 = sin(angle + 8*PI/5) * 100 + height/2;
// 绘制星形
beginShape();
vertex(x1, y1);
vertex(x3, y3);
vertex(x5, y5);
vertex(x2, y2);
vertex(x4, y4);
endShape(CLOSE);
// 增加角度
angle += 0.05;
}
```
5. 绘制圆形波浪线
```java
float angle = 0;
void setup() {
size(400, 400);
smooth();
}
void draw() {
background(255);
// 计算圆形波浪线的坐标
float x = cos(angle) * (50 + 10 * sin(angle)) + width/2;
float y = sin(angle) * (50 + 10 * sin(angle)) + height/2;
// 绘制圆形
ellipse(x, y, 5, 5);
// 增加角度
angle += 0.05;
}
```
6. 绘制蝴蝶线
```java
float angle = 0;
void setup() {
size(400, 400);
smooth();
}
void draw() {
background(255);
// 计算蝴蝶线的坐标
float x = sin(angle) * (exp(cos(angle)) - 2*cos(4*angle) - pow(sin(angle/12), 5)) * 80 + width/2;
float y = cos(angle) * (exp(cos(angle)) - 2*cos(4*angle) - pow(sin(angle/12), 5)) * 80 + height/2;
// 绘制圆形
ellipse(x, y, 5, 5);
// 增加角度
angle += 0.05;
}
```
7. 绘制花瓣形状
```java
float angle = 0;
void setup() {
size(400, 400);
smooth();
}
void draw() {
background(255);
// 计算花瓣形状的坐标
float x = sin(angle) * (5 * cos(angle/4) + 5) + width/2;
float y = cos(angle) * (5 * cos(angle/4) + 5) + height/2;
// 绘制圆形
ellipse(x, y, 5, 5);
// 增加角度
angle += 0.05;
}
```
8. 绘制螺线
```java
float angle = 0;
float radius = 10;
void setup() {
size(400, 400);
smooth();
}
void draw() {
background(255);
// 计算螺线的坐标
float x = cos(angle) * radius + width/2;
float y = sin(angle) * radius + height/2;
// 绘制圆形
ellipse(x, y, 5, 5);
// 增加角度和半径
angle += 0.1;
radius += 0.05;
}
```
9. 绘制摆线
```java
float angle = 0;
void setup() {
size(400, 400);
smooth();
}
void draw() {
background(255);
// 计算摆线的坐标
float x = sin(angle) * 100;
float y = cos(angle) * 100 + sin(angle) * 50;
x = x + width/2;
y = y + height/2;
// 绘制圆形
ellipse(x, y, 5, 5);
// 增加角度
angle += 0.05;
}
```
10. 绘制正弦曲线
```java
float angle = 0;
void setup() {
size(400, 400);
smooth();
}
void draw() {
background(255);
// 计算正弦曲线的坐标
float x = angle;
float y = sin(angle) * 100 + height/2;
// 绘制圆形
ellipse(x, y, 5, 5);
// 增加角度
angle += 0.05;
}
```
以上是十个使用三角函数创建动画的 Processing 代码示例,你可以将其复制到 Processing 编辑器中运行并观察效果。
阅读全文
相关推荐
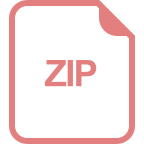
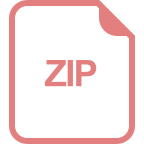
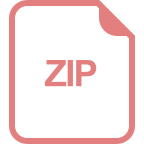














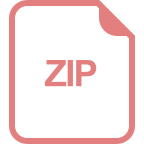
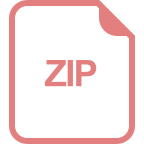