在c#窗体文件中用picturebox如何实现视频的稠密光流法跟踪,即在picturebox中播放视频,在视频中有稠密光流法跟踪(步骤包含所用控件名称,如何得到该控件,代码实现视频的稠密光流法跟踪),所用CalcOpticalFlowFarneback方法只有10个参数,用的是opencv中的知识点
时间: 2023-12-09 16:05:15 浏览: 303
实现视频的稠密光流法跟踪需要以下步骤:
1. 创建一个PictureBox控件来显示视频帧。
2. 导入OpenCV库。
3. 打开视频文件,使用VideoCapture类来读取每一帧。
4. 对每一帧应用稠密光流法跟踪算法,使用CalcOpticalFlowFarneback方法来计算光流向量。
5. 为了可视化光流向量,可以在每一帧上绘制箭头来表示光流方向和大小。
6. 将处理后的帧显示在PictureBox控件中。
以下是实现视频的稠密光流法跟踪的C#代码示例:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using OpenCvSharp;
namespace DenseOpticalFlowDemo
{
public partial class Form1 : Form
{
private VideoCapture _capture;
private Mat _previousFrame;
private Mat _currentFrame;
private Mat _outputFrame;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
// 打开视频文件
_capture = new VideoCapture("test.mp4");
if (!_capture.IsOpened())
{
MessageBox.Show("Failed to open video file!");
Close();
return;
}
// 读取第一帧并转换为灰度图像
_capture.Read(_previousFrame);
Cv2.CvtColor(_previousFrame, _previousFrame, ColorConversionCodes.BGR2GRAY);
// 创建输出帧
_outputFrame = new Mat(_previousFrame.Rows, _previousFrame.Cols, MatType.CV_8UC3);
}
private void timer1_Tick(object sender, EventArgs e)
{
// 读取下一帧并转换为灰度图像
_capture.Read(_currentFrame);
Cv2.CvtColor(_currentFrame, _currentFrame, ColorConversionCodes.BGR2GRAY);
// 计算稠密光流向量
var flow = new Mat();
Cv2.CalcOpticalFlowFarneback(_previousFrame, _currentFrame, flow, 0.5, 3, 15, 3, 5, 1.2, 0);
// 可视化光流向量
for (int y = 0; y < _outputFrame.Rows; y += 10)
{
for (int x = 0; x < _outputFrame.Cols; x += 10)
{
var flowVec = flow.At<Point2f>(y, x);
Cv2.Line(_outputFrame, new Point(x, y), new Point((int)(x + flowVec.X), (int)(y + flowVec.Y)), Scalar.Red, 2);
Cv2.Circle(_outputFrame, new Point(x, y), 2, Scalar.Blue, 2);
}
}
// 将处理后的帧显示在PictureBox中
pictureBox1.Image = BitmapConverter.ToBitmap(_outputFrame);
// 更新上一帧为当前帧
_previousFrame = _currentFrame.Clone();
}
}
}
```
在上述代码中,我们使用了一个Windows Forms应用程序,其中包含一个PictureBox控件和一个计时器控件。在窗体加载时,我们打开视频文件并读取第一帧作为上一帧,以便在下一次计时器事件时使用。在计时器事件中,我们读取下一帧并计算稠密光流向量,然后在每个像素位置绘制箭头来表示光流方向和大小。最后,我们将处理后的帧显示在PictureBox控件中,更新上一帧为当前帧。
请注意,稠密光流法跟踪是一项计算密集型任务,可能需要较长时间才能处理完整个视频。为了避免在UI线程上进行长时间计算,建议将计算过程放在单独的线程中执行,并使用Invoke方法在UI线程上更新PictureBox控件。
阅读全文
相关推荐
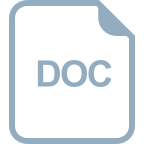
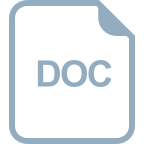
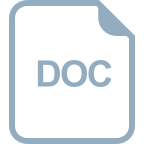

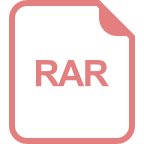
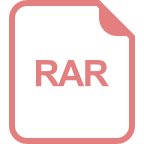
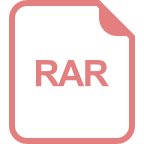
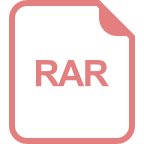
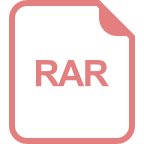
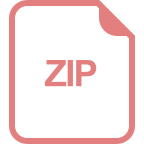
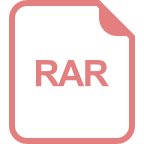
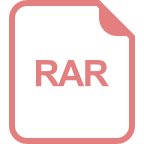
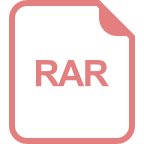
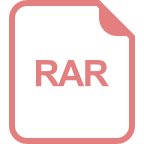
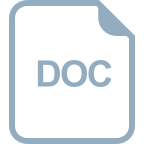


