在c#窗体文件中用picturebox如何实现视频的光流法跟踪,即在picturebox中播放视频,在视频中有光流法跟踪,用的是opencv中的知识点,点击按钮自定义选择视频文件,写出代码和解释确保可以正常运行
时间: 2023-12-09 10:05:16 浏览: 260
实现视频光流法跟踪需要用到OpenCV库,可以使用Emgu CV库来在C#中调用OpenCV。
首先,需要安装Emgu CV库并将其添加到Visual Studio项目中。
然后,我们需要使用VideoCapture类来读取视频文件并将每一帧显示在PictureBox中。接着,我们可以使用OpenCV中的光流法函数来跟踪视频中的运动。
下面是一个示例代码,实现了视频光流法跟踪:
```csharp
using Emgu.CV;
using Emgu.CV.CvEnum;
using Emgu.CV.Structure;
using Emgu.CV.Util;
using System;
using System.Drawing;
using System.Windows.Forms;
namespace VideoOpticalFlow
{
public partial class MainForm : Form
{
private VideoCapture _capture;
private Mat _previousFrame;
public MainForm()
{
InitializeComponent();
}
private void btnLoadVideo_Click(object sender, EventArgs e)
{
OpenFileDialog openFileDialog = new OpenFileDialog();
openFileDialog.Filter = "Video files (*.avi;*.mp4)|*.avi;*.mp4";
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
_capture = new VideoCapture(openFileDialog.FileName);
_capture.ImageGrabbed += ProcessFrame;
_capture.Start();
}
}
private void ProcessFrame(object sender, EventArgs e)
{
Mat frame = new Mat();
_capture.Retrieve(frame, 0);
if (_previousFrame == null)
{
_previousFrame = frame.Clone();
return;
}
Mat grayPrevious = new Mat();
Mat grayCurrent = new Mat();
CvInvoke.CvtColor(_previousFrame, grayPrevious, ColorConversion.Bgr2Gray);
CvInvoke.CvtColor(frame, grayCurrent, ColorConversion.Bgr2Gray);
VectorOfPointF previousPoints = new VectorOfPointF();
CvInvoke.GoodFeaturesToTrack(grayPrevious, previousPoints, 500, 0.01, 10);
VectorOfPointF currentPoints = new VectorOfPointF();
VectorOfByte status = new VectorOfByte();
VectorOfFloat error = new VectorOfFloat();
CvInvoke.CalcOpticalFlowPyrLK(grayPrevious, grayCurrent, previousPoints, currentPoints, status, error);
for (int i = 0; i < currentPoints.Size; i++)
{
PointF previousPoint = previousPoints[i];
PointF currentPoint = currentPoints[i];
if (status[i] == 1 && error[i] < 15)
{
CvInvoke.Line(frame, Point.Round(previousPoint), Point.Round(currentPoint), new Bgr(Color.Red).MCvScalar, 2);
CvInvoke.Circle(frame, Point.Round(currentPoint), 3, new Bgr(Color.Blue).MCvScalar, -1);
}
}
_previousFrame = frame.Clone();
pictureBox.Image = frame.Bitmap;
}
private void MainForm_FormClosing(object sender, FormClosingEventArgs e)
{
if (_capture != null)
{
_capture.Stop();
}
}
}
}
```
在这个示例代码中,我们使用`VideoCapture`类来读取视频文件,并在`ProcessFrame`方法中处理每一帧。在`ProcessFrame`方法中,我们首先将当前帧和上一帧转换为灰度图像,并使用`GoodFeaturesToTrack`函数找到前一帧中的特征点。接着,我们使用`CalcOpticalFlowPyrLK`函数计算两帧之间的光流,并在图像中绘制运动跟踪的线和点。最后,我们将处理后的图像显示在`PictureBox`中。
在这个示例代码中,我们还添加了一个按钮来选择视频文件,并在窗体关闭时停止视频捕获。
请注意,这个示例代码只是一个简单的示例,如果要进行更复杂的运动跟踪,需要更多的OpenCV知识和技能。
阅读全文
相关推荐
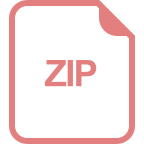
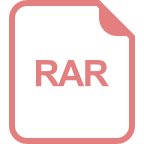









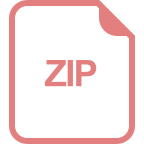
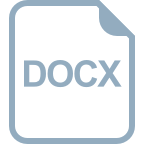