libusb打开USB设备的示例
时间: 2023-06-07 09:09:32 浏览: 217
以下是libusb打开USB设备的示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <libusb.h>
#define LOGD(...) printf(__VA_ARGS__)
#define LOGE(...) fprintf(stderr, __VA_ARGS__)
#define VENDOR_ID 0x04f2 // Example vendor ID
#define PRODUCT_ID 0xb40 // Example product ID
static int find_device(libusb_device **dev) {
libusb_device **devs;
ssize_t cnt = libusb_get_device_list(NULL, &devs);
if (cnt < 0) {
LOGE("Failed to get device list!\n");
return cnt;
}
for (ssize_t i = 0; i < cnt; ++i) {
struct libusb_device_descriptor desc;
int ret;
ret = libusb_get_device_descriptor(devs[i], &desc);
if (ret < 0) {
continue;
}
if (desc.idVendor == VENDOR_ID && desc.idProduct == PRODUCT_ID) {
*dev = devs[i];
free(devs);
return 1;
}
}
LOGE("No device found!\n");
free(devs);
return 0;
}
int main() {
libusb_context *ctx = NULL;
libusb_device *dev = NULL;
int ret;
// Initialize libusb
ret = libusb_init(&ctx);
if (ret < 0) {
LOGE("Failed to initialize libusb!\n");
return EXIT_FAILURE;
}
ret = find_device(&dev);
if (ret <= 0) {
libusb_exit(ctx);
return EXIT_FAILURE;
}
libusb_device_handle *handle;
ret = libusb_open(dev, &handle);
if (ret < 0) {
LOGE("Failed to open device!\n");
libusb_exit(ctx);
return EXIT_FAILURE;
}
// Do something with the device here...
libusb_close(handle);
libusb_exit(ctx);
return EXIT_SUCCESS;
}
```
阅读全文
相关推荐
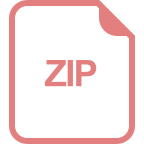
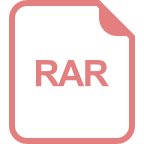
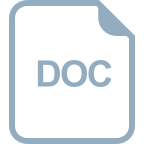
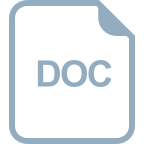


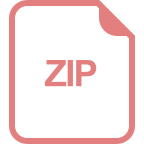
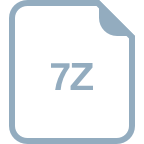
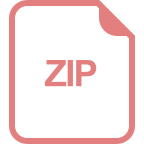
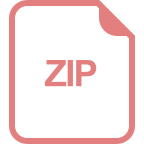
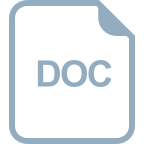
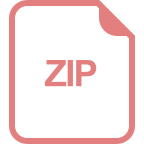
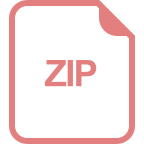
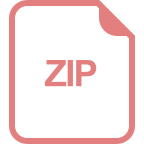
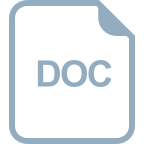

