编程实现一个简单的大学人员管理程序,该程序可以管理大学的一些基本人员:学生、教师、职工。首先设计一个抽象类person。通过该类保存人员的最基本信息:姓名 (name)、年龄(age)和性别 (sex),同时定义一个静态数据成员用来统计人数(sum)。然后使用该类派生出学生类、教师类和职工类,在其中添加各自的特性,如在学生类中添加如下信息:专业(speciality) 在教师类中添加院系(department),在职工类中添加部门和职务等信息。为每个类定义一个输出函数print0,输出该类相关信;定义一个保存函数保存相关信息到文件
时间: 2024-03-14 11:46:58 浏览: 116
好的,我可以帮你解答这个问题。
首先,我们需要定义一个抽象类`person`,其中包含`name`、`age`和`sex`三个成员变量和一个静态数据成员`sum`。代码如下:
```cpp
#include <string>
class person {
public:
person(const std::string& name_, int age_, const std::string& sex_)
: name(name_), age(age_), sex(sex_) {
++sum;
}
virtual ~person() {}
virtual void print() const = 0;
static int sum;
protected:
std::string name;
int age;
std::string sex;
};
int person::sum = 0;
```
在这个基类中,我们定义了一个纯虚函数`print()`,用于输出相关信息。同时,我们定义了一个静态数据成员`sum`,用于统计人数。
接下来,我们可以使用该类派生出学生类、教师类和职工类,并分别添加各自的特性。代码如下:
```cpp
#include <iostream>
#include <fstream>
class student : public person {
public:
student(const std::string& name_, int age_, const std::string& sex_,
const std::string& speciality_)
: person(name_, age_, sex_), speciality(speciality_) {}
void print() const override {
std::cout << "Name: " << name << ", Age: " << age << ", Sex: " << sex
<< ", Speciality: " << speciality << std::endl;
}
void save(std::ofstream& ofs) const {
ofs << "student " << name << " " << age << " " << sex << " " << speciality << std::endl;
}
private:
std::string speciality;
};
class teacher : public person {
public:
teacher(const std::string& name_, int age_, const std::string& sex_,
const std::string& department_)
: person(name_, age_, sex_), department(department_) {}
void print() const override {
std::cout << "Name: " << name << ", Age: " << age << ", Sex: " << sex
<< ", Department: " << department << std::endl;
}
void save(std::ofstream& ofs) const {
ofs << "teacher " << name << " " << age << " " << sex << " " << department << std::endl;
}
private:
std::string department;
};
class staff : public person {
public:
staff(const std::string& name_, int age_, const std::string& sex_,
const std::string& department_, const std::string& position_)
: person(name_, age_, sex_), department(department_), position(position_) {}
void print() const override {
std::cout << "Name: " << name << ", Age: " << age << ", Sex: " << sex
<< ", Department: " << department << ", Position: " << position << std::endl;
}
void save(std::ofstream& ofs) const {
ofs << "staff " << name << " " << age << " " << sex << " " << department << " " << position << std::endl;
}
private:
std::string department;
std::string position;
};
```
在这些派生类中,我们重载了基类中的`print()`函数,并添加了一个新的成员函数`save()`,用于将相关信息保存到文件中。
最后,我们可以编写一个`main()`函数来测试这些类的功能。在主函数中,我们创建了一些学生、教师和职工对象,并调用它们的`print()`函数和`save()`函数。代码如下:
```cpp
#include <vector>
int main() {
std::vector<person*> people;
people.push_back(new student("Tom", 20, "Male", "Computer Science"));
people.push_back(new teacher("Jack", 35, "Male", "Computer Science"));
people.push_back(new staff("Mary", 25, "Female", "Administration", "Secretary"));
for (auto p : people) {
p->print();
}
std::ofstream ofs("people.txt");
for (auto p : people) {
p->save(ofs);
}
return 0;
}
```
这个程序将输出以下内容:
```
Name: Tom, Age: 20, Sex: Male, Speciality: Computer Science
Name: Jack, Age: 35, Sex: Male, Department: Computer Science
Name: Mary, Age: 25, Sex: Female, Department: Administration, Position: Secretary
```
并且将把这些人员的信息保存到`people.txt`文件中。文件内容如下:
```
student Tom 20 Male Computer Science
teacher Jack 35 Male Computer Science
staff Mary 25 Female Administration Secretary
```
这样,我们就完成了一个简单的大学人员管理程序。
阅读全文
相关推荐





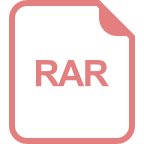










