3.编程实现一个简单的大学人员管理程序,该程序可以管理大学的一些基本人员:学生、教师、职工。首先设计一个抽象类person。通过该类保存人员的最基本信息:姓名(name)、年龄(age)和性别(sex),同时定义一个静态数据成员用来统计人数(sum)。然后使用该类派生出学生类、教师类和职工类,在其中添加各自的特性,如在学生类中添加如下信息:专业(speciality),在教师类中添加院系(department),在职工类中添加部门和职务等信息。为每个类定义一个输出函数print(),输出该类相关信;定义一个保存函数保存相关信息到文件。
时间: 2023-07-16 08:17:13 浏览: 138
以下是一个基本的大学人员管理程序的实现:
```C++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Person {
public:
Person(string n, int a, string s) : name(n), age(a), sex(s) {
sum++;
}
virtual void print() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Sex: " << sex << endl;
}
static int getSum() {
return sum;
}
protected:
string name;
int age;
string sex;
static int sum;
};
class Student : public Person {
public:
Student(string n, int a, string s, string spec) : Person(n, a, s), speciality(spec) {}
void print() {
Person::print();
cout << "Speciality: " << speciality << endl;
}
void saveToFile() {
ofstream file("student.txt");
file << "Name: " << name << endl;
file << "Age: " << age << endl;
file << "Sex: " << sex << endl;
file << "Speciality: " << speciality << endl;
file.close();
}
private:
string speciality;
};
class Teacher : public Person {
public:
Teacher(string n, int a, string s, string dept) : Person(n, a, s), department(dept) {}
void print() {
Person::print();
cout << "Department: " << department << endl;
}
void saveToFile() {
ofstream file("teacher.txt");
file << "Name: " << name << endl;
file << "Age: " << age << endl;
file << "Sex: " << sex << endl;
file << "Department: " << department << endl;
file.close();
}
private:
string department;
};
class Staff : public Person {
public:
Staff(string n, int a, string s, string dept, string position) : Person(n, a, s), department(dept), position(position) {}
void print() {
Person::print();
cout << "Department: " << department << endl;
cout << "Position: " << position << endl;
}
void saveToFile() {
ofstream file("staff.txt");
file << "Name: " << name << endl;
file << "Age: " << age << endl;
file << "Sex: " << sex << endl;
file << "Department: " << department << endl;
file << "Position: " << position << endl;
file.close();
}
private:
string department;
string position;
};
int Person::sum = 0;
int main() {
Student s("Tom", 20, "Male", "Computer Science");
Teacher t("Jack", 35, "Male", "Computer Science");
Staff st("Lucy", 30, "Female", "Administration", "Secretary");
s.print();
s.saveToFile();
cout << endl;
t.print();
t.saveToFile();
cout << endl;
st.print();
st.saveToFile();
cout << endl;
cout << "Total number of people: " << Person::getSum() << endl;
return 0;
}
```
在这个程序中,我们首先定义了一个抽象类 `Person`,其中包含了人员的最基本信息:姓名、年龄和性别,并声明了一个静态数据成员 `sum`,用于统计人数。接下来,我们定义了 `Student`、`Teacher` 和 `Staff` 三个类,它们分别继承自 `Person`,并在其中添加了各自的特性。每个类都有一个输出函数 `print()`,用于输出该类相关信息;以及一个保存函数 `saveToFile()`,用于将相关信息保存到文件中。
在 `main()` 函数中,我们创建了一个学生、一个教师和一个职工,并分别调用它们的 `print()` 和 `saveToFile()` 函数。最后,我们输出了总人数。
注意,在这里我们使用了 C++11 的初始化列表语法来对基类的成员进行初始化。同时,为了方便起见,我们在每个类的保存函数中都使用了 ofstream 类来将信息保存到文件中,而没有使用更加复杂的文件流操作。
阅读全文
相关推荐


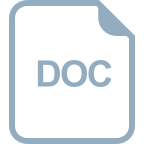
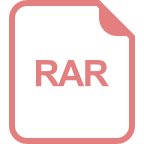
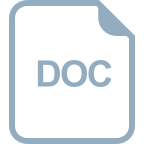
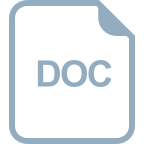
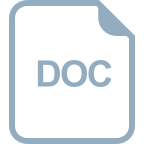
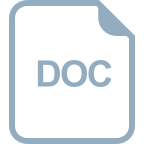
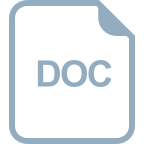
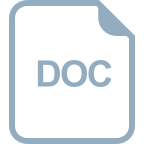
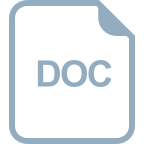
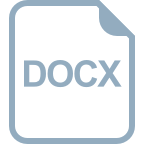
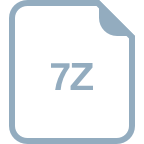
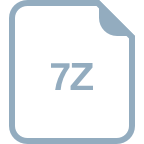
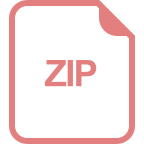