编程实现一个简单的大学人员管理程序,该程序可以管理大学的一些基本人员:学生、教师、职工。首先设计一个抽象类person。通过该类保存人员的最基本信息:姓名 (name)、年龄(age)和性别 (sex),同时定义一个静态数据成员用来统计人数(sum)。然后使用该类派生出学生类、教师类和职工类,在其中添加各自的特性,如在学生类中添加如下信息:专业(speciality)在教师类中添加院系 (department),在职工类中添加部门和职务等信息。为每个类定义一个输出函数print0,输出该类相关信;定义一个保存函数保存相关信息到文件
时间: 2024-03-16 21:47:43 浏览: 84
好的,我会根据您的要求来回答问题。对于您的问题,我可以提供以下代码示例:
```python
import pickle
class Person:
sum = 0
def __init__(self, name, age, sex):
self.name = name
self.age = age
self.sex = sex
Person.sum += 1
@staticmethod
def get_sum():
return Person.sum
def print_info(self):
print("Name:", self.name)
print("Age:", self.age)
print("Sex:", self.sex)
class Student(Person):
def __init__(self, name, age, sex, speciality):
super().__init__(name, age, sex)
self.speciality = speciality
def print_info(self):
super().print_info()
print("Speciality:", self.speciality)
def save_info(self):
with open('students.pickle', 'ab') as f:
pickle.dump(self, f)
class Teacher(Person):
def __init__(self, name, age, sex, department):
super().__init__(name, age, sex)
self.department = department
def print_info(self):
super().print_info()
print("Department:", self.department)
def save_info(self):
with open('teachers.pickle', 'ab') as f:
pickle.dump(self, f)
class Staff(Person):
def __init__(self, name, age, sex, department, position):
super().__init__(name, age, sex)
self.department = department
self.position = position
def print_info(self):
super().print_info()
print("Department:", self.department)
print("Position:", self.position)
def save_info(self):
with open('staff.pickle', 'ab') as f:
pickle.dump(self, f)
# 测试代码
s = Student("Tom", 20, "male", "Computer Science")
t = Teacher("Kate", 35, "female", "Mathematics")
st = Staff("Jack", 25, "male", "Human Resources", "Manager")
s.print_info()
print()
t.print_info()
print()
st.print_info()
print()
s.save_info()
t.save_info()
st.save_info()
print("Total people:", Person.get_sum())
```
此代码定义了一个 `Person` 抽象类,以及它的三个子类 `Student`、`Teacher` 和 `Staff`。每个子类都添加了一些特定的信息,并且都有一个输出函数 `print_info`,可以输出该类相关信息。此外,每个子类还有一个保存函数 `save_info`,可以将相关信息保存到文件中(使用了 `pickle` 模块来进行序列化和反序列化操作)。
在测试代码中,我们创建了一个学生、一个教师和一个职工,分别输出它们的信息并将它们保存到文件中。最后,我们还可以通过调用 `Person.get_sum()` 函数来获取创建的人员总数。
阅读全文
相关推荐
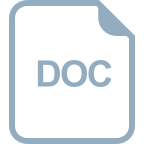
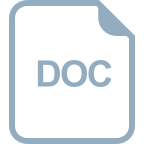
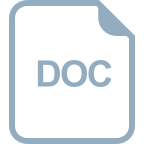


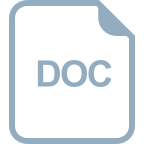
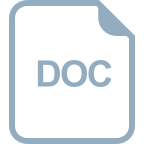
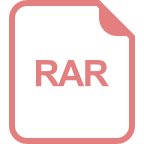
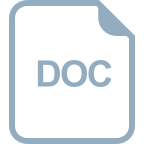
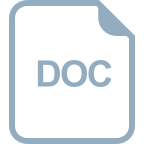
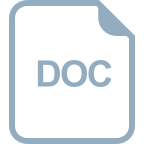
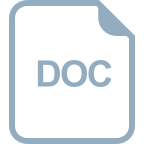
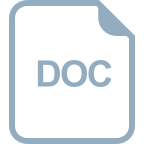
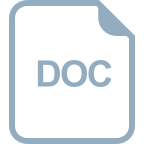
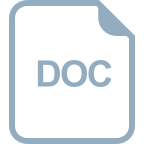
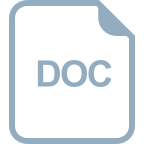
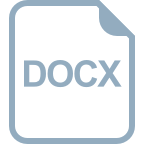