用c语言写一个歌曲管理系统
时间: 2023-11-11 10:07:46 浏览: 122
好的,下面是一个简单的歌曲管理系统的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SONGS 100
struct Song {
char title[100];
char artist[100];
int year;
};
struct Song song_list[MAX_SONGS];
int num_songs = 0;
void add_song() {
if (num_songs == MAX_SONGS) {
printf("Unable to add song: list is full.\n");
return;
}
struct Song new_song;
printf("Title: ");
fgets(new_song.title, sizeof(new_song.title), stdin);
new_song.title[strcspn(new_song.title, "\n")] = '\0';
printf("Artist: ");
fgets(new_song.artist, sizeof(new_song.artist), stdin);
new_song.artist[strcspn(new_song.artist, "\n")] = '\0';
printf("Year: ");
scanf("%d", &new_song.year);
getchar(); // consume newline character left over from scanf
song_list[num_songs] = new_song;
num_songs++;
printf("Song added.\n");
}
void list_songs() {
if (num_songs == 0) {
printf("No songs in list.\n");
return;
}
printf("Title\tArtist\tYear\n");
for (int i = 0; i < num_songs; i++) {
printf("%s\t%s\t%d\n", song_list[i].title, song_list[i].artist, song_list[i].year);
}
}
int main() {
while (1) {
printf("Enter a command (add, list, quit): ");
char command[10];
fgets(command, sizeof(command), stdin);
command[strcspn(command, "\n")] = '\0';
if (strcmp(command, "add") == 0) {
add_song();
} else if (strcmp(command, "list") == 0) {
list_songs();
} else if (strcmp(command, "quit") == 0) {
break;
} else {
printf("Invalid command.\n");
}
}
return 0;
}
```
这个程序使用结构体 `Song` 来存储每首歌曲的信息,包括标题、艺术家和年份。歌曲列表存储在全局数组 `song_list` 中,最多可以存储 `MAX_SONGS` 首歌曲。程序提供了三个命令:`add` 用于添加新歌曲,`list` 用于列出所有歌曲,`quit` 用于退出程序。在添加新歌曲时,程序会提示用户输入歌曲标题、艺术家和年份,并将新歌曲添加到列表中。在列出所有歌曲时,程序会遍历列表并输出每首歌曲的信息。
阅读全文
相关推荐
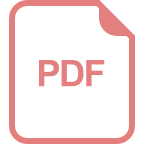
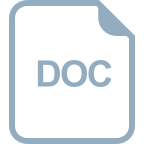

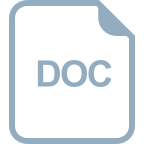
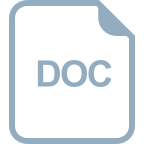
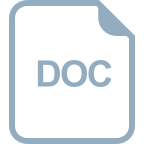
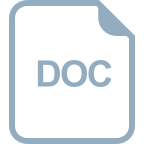
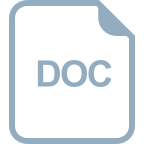
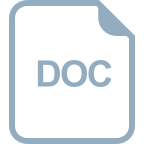
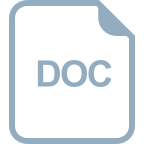
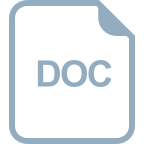
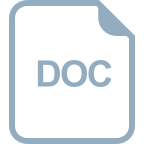
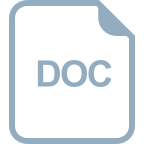


