歌曲信息管理系统c语言代码
时间: 2023-12-28 09:02:05 浏览: 339
歌曲信息管理系统是一个用于管理歌曲信息的软件系统,用户可以通过该系统添加、删除、查找和修改歌曲信息。以下是一个简单的用C语言编写的歌曲信息管理系统的代码示例:
```c
#include <stdio.h>
#include <string.h>
#define MAX_SONGS 100
struct Song {
char title[100];
char artist[100];
char album[100];
int year;
};
struct Song songs[MAX_SONGS];
int count = 0;
void addSong(char title[], char artist[], char album[], int year) {
if (count < MAX_SONGS) {
strcpy(songs[count].title, title);
strcpy(songs[count].artist, artist);
strcpy(songs[count].album, album);
songs[count].year = year;
count++;
printf("歌曲信息添加成功!\n");
} else {
printf("歌曲信息库已满,无法添加新歌曲。\n");
}
}
void displaySongs() {
if (count > 0) {
printf("歌曲信息列表:\n");
for (int i = 0; i < count; i++) {
printf("标题:%s, 艺术家:%s, 专辑:%s, 年份:%d\n", songs[i].title, songs[i].artist, songs[i].album, songs[i].year);
}
} else {
printf("歌曲信息库为空。\n");
}
}
void deleteSong(char title[]) {
int found = 0;
for (int i = 0; i < count; i++) {
if (strcmp(songs[i].title, title) == 0) {
for (int j = i; j < count - 1; j++) {
strcpy(songs[j].title, songs[j + 1].title);
strcpy(songs[j].artist, songs[j + 1].artist);
strcpy(songs[j].album, songs[j + 1].album);
songs[j].year = songs[j + 1].year;
}
count--;
found = 1;
printf("歌曲信息删除成功!\n");
}
}
if (!found) {
printf("未找到该歌曲信息。\n");
}
}
int main() {
addSong("夜曲", "周杰伦", "十一月的萧邦", 2005);
addSong("告白气球", "周杰伦", "周杰伦的床边故事", 2016);
displaySongs();
deleteSong("夜曲");
displaySongs();
return 0;
}
```
以上是一个简单的歌曲信息管理系统的C语言代码示例,包括了添加歌曲、显示歌曲列表和删除歌曲信息的功能。当然,实际的歌曲信息管理系统会更加复杂和完善,包括更多的功能和用户界面设计。
阅读全文
相关推荐
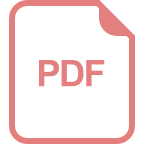
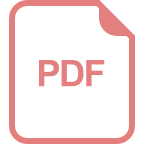
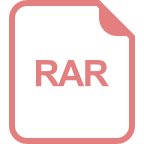
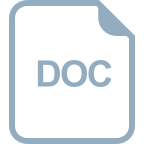
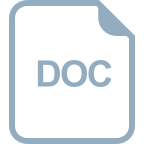
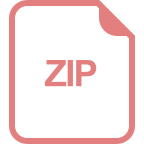
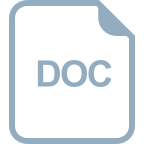
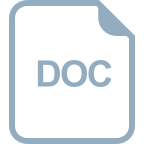
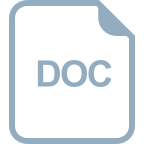
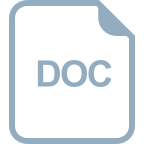
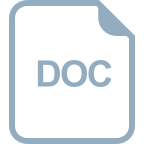
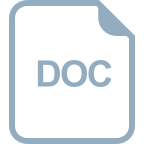
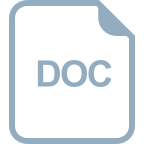

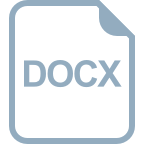