用c++写输入3个数,求最大值的程序
时间: 2023-03-31 18:03:45 浏览: 65
您好,以下是用 C 语言编写输入 3 个数,求最大值的程序:
#include <stdio.h>
int main() {
int a, b, c, max;
printf("请输入三个数,用空格隔开:");
scanf("%d %d %d", &a, &b, &c);
max = a;
if (b > max) {
max = b;
}
if (c > max) {
max = c;
}
printf("最大值为:%d\n", max);
return ;
}
希望能对您有所帮助。
相关问题
c++输入10个数输出最大值
好的,可以使用以下的代码实现:
```c++
#include <iostream>
using namespace std;
int main() {
int nums[10];
int max_num = INT_MIN; // 初始化最大值为int类型的最小值
cout << "请输入10个数:" << endl;
for (int i = 0; i < 10; i++) {
cin >> nums[i];
if (nums[i] > max_num) {
max_num = nums[i];
}
}
cout << "最大值为:" << max_num << endl;
return 0;
}
```
程序中使用了一个数组 `nums` 存储用户输入的10个数,然后使用一个变量 `max_num` 来记录最大值。在输入每个数时,都与 `max_num` 进行比较,如果大于 `max_num` 则更新 `max_num`。最后输出 `max_num` 即可。
使用C++输入20个数,求其最大、最小和平均值
好的。
在 C 语言中,要输入 20 个数,求其最大、最小和平均值,可以这样做:
1. 在程序中声明三个变量,分别用来存储最大值、最小值和平均值,例如:
```
int max, min, sum;
float avg;
```
2. 使用循环语句,依次输入 20 个数,并在输入过程中进行判断。每次输入一个数时,将该数和当前的最大值进行比较,如果该数大于最大值,就将最大值更新为该数;将该数和当前的最小值进行比较,如果该数小于最小值,就将最小值更新为该数。同时,将所有输入的数累加到变量 sum 中。
3. 在循环结束后,计算平均值,即将 sum 除以 20 得到的结果。
4. 最后,输出最大值、最小值和平均值。
下面是一个例子:
```
#include <stdio.h>
int main()
{
int max, min, sum;
float avg;
int i, num;
printf("输入 20 个数:\n");
// 输入第一个数,并将其赋值给 max 和 min
scanf("%d", &num);
max = min = num;
sum = num;
// 从第二个数开始输入
for (i = 2; i <= 20; i++) {
scanf("%d", &num);
// 比较并更新最大值和
相关推荐
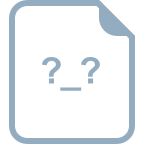
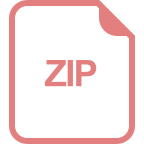
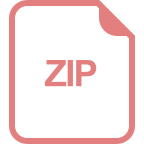












