js编写一个简单的轮播图,带箭头和圆点
时间: 2024-02-15 12:02:14 浏览: 70
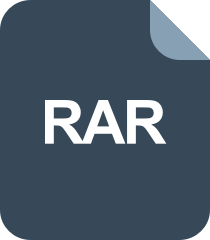
简单的js轮播图
以下是一个简单的轮播图的HTML和JavaScript代码,带有箭头和圆点:
```html
<!DOCTYPE html>
<html>
<head>
<title>轮播图</title>
<style>
#carousel {
position: relative;
overflow: hidden;
height: 400px;
width: 800px;
margin: 0 auto;
}
#carousel img {
position: absolute;
top: 0;
left: 0;
height: 400px;
width: 800px;
opacity: 0;
transition: opacity 1s;
}
#carousel img.active {
opacity: 1;
}
#carousel .arrow {
position: absolute;
top: 50%;
transform: translateY(-50%);
cursor: pointer;
font-size: 3em;
color: #fff;
background-color: rgba(0, 0, 0, 0.5);
padding: 0.5em;
border-radius: 5px;
z-index: 1;
}
#carousel .arrow.prev {
left: 0;
}
#carousel .arrow.next {
right: 0;
}
#carousel .dots {
position: absolute;
bottom: 0;
left: 50%;
transform: translateX(-50%);
display: flex;
flex-direction: row;
align-items: center;
justify-content: center;
margin-bottom: 1em;
}
#carousel .dot {
height: 0.5em;
width: 0.5em;
margin: 0 0.2em;
border-radius: 50%;
background-color: rgba(255, 255, 255, 0.5);
cursor: pointer;
transition: background-color 0.5s;
}
#carousel .dot.active {
background-color: #fff;
}
</style>
</head>
<body>
<div id="carousel">
<img src="img1.jpg" class="active">
<img src="img2.jpg">
<img src="img3.jpg">
<div class="arrow prev">‹</div>
<div class="arrow next">›</div>
<div class="dots">
<div class="dot active"></div>
<div class="dot"></div>
<div class="dot"></div>
</div>
</div>
<script>
const carousel = document.getElementById('carousel');
const images = carousel.querySelectorAll('img');
const arrows = carousel.querySelectorAll('.arrow');
const dots = carousel.querySelectorAll('.dot');
let index = 0;
function changeImage() {
images.forEach(img => img.classList.remove('active'));
images[index].classList.add('active');
dots.forEach(dot => dot.classList.remove('active'));
dots[index].classList.add('active');
}
function nextImage() {
index = (index + 1) % images.length;
changeImage();
}
function prevImage() {
index = (index + images.length - 1) % images.length;
changeImage();
}
function dotClicked(e) {
index = Array.prototype.indexOf.call(dots, e.target);
changeImage();
}
arrows.forEach(arrow => arrow.addEventListener('click', e => {
if (e.target.classList.contains('prev')) {
prevImage();
} else {
nextImage();
}
}));
dots.forEach(dot => dot.addEventListener('click', dotClicked));
</script>
</body>
</html>
```
在这个代码中,我们使用了HTML的`<img>`标签来显示图片,CSS来布局和样式化轮播图,以及JavaScript来实现轮播图的逻辑。我们在HTML中定义了轮播图的容器`<div id="carousel">`,其中包含了图片、箭头和圆点等元素。我们为容器设置了宽度和高度,并使用`overflow: hidden`属性来隐藏超出容器范围的元素。我们在CSS中使用绝对定位和`opacity`属性来实现轮播图的切换效果,使用`transition`属性来实现渐变动画。我们使用JavaScript来实现图片切换、箭头和圆点点击等交互逻辑。我们在JavaScript中定义了`changeImage()`函数来切换图片和圆点的状态,定义了`nextImage()`和`prevImage()`函数来切换下一张和上一张图片,定义了`dotClicked()`函数来处理圆点的点击事件。我们使用`addEventListener()`方法来为箭头和圆点添加点击事件监听器,使用`classList`属性来添加或移除CSS类来改变元素的状态。
阅读全文
相关推荐
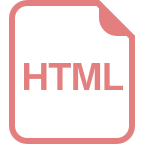
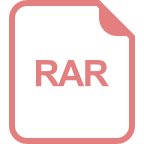

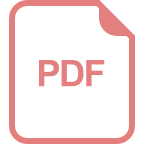
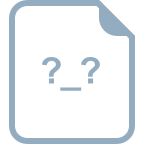
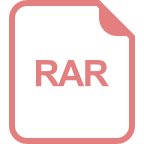
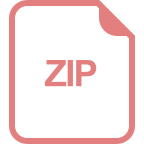
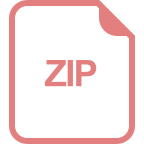
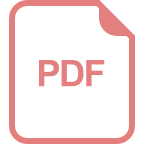
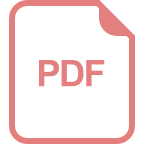
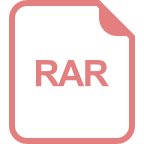
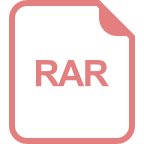
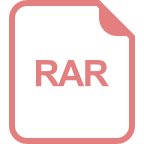
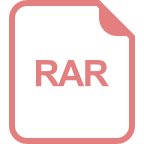
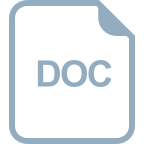
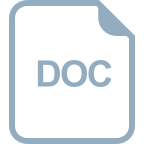
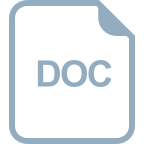
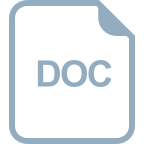