linux posix_spawn 创建不同文件夹下的进程
时间: 2023-11-22 12:54:59 浏览: 38
可以使用 `chdir` 函数改变子进程的工作目录,然后再使用 `posix_spawn` 函数创建子进程。
下面是一个示例代码,用于在不同的文件夹下创建子进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <spawn.h>
#include <string.h>
int main(int argc, char *argv[]) {
pid_t pid;
char *params[] = {"ls", "-l", NULL};
posix_spawn_file_actions_t actions;
posix_spawn_file_actions_init(&actions);
char *path1 = "/path/to/directory1";
char *path2 = "/path/to/directory2";
if ((pid = fork()) == 0) {
// Child process
chdir(path1);
posix_spawn_file_actions_addchdir(&actions, path1);
posix_spawn(NULL, "/bin/ls", &actions, NULL, params, NULL);
exit(0);
} else if (pid > 0) {
// Parent process
waitpid(pid, NULL, 0);
} else {
perror("fork failed");
exit(1);
}
if ((pid = fork()) == 0) {
// Child process
chdir(path2);
posix_spawn_file_actions_addchdir(&actions, path2);
posix_spawn(NULL, "/bin/ls", &actions, NULL, params, NULL);
exit(0);
} else if (pid > 0) {
// Parent process
waitpid(pid, NULL, 0);
} else {
perror("fork failed");
exit(1);
}
posix_spawn_file_actions_destroy(&actions);
return 0;
}
```
在上面的代码中,我们通过 `chdir` 函数改变了子进程的工作目录,并通过 `posix_spawn_file_actions_addchdir` 函数将该目录添加到 `posix_spawn_file_actions_t` 结构体中,以便在创建子进程时使用。这样,在子进程中调用 `ls` 命令就会在指定的目录中执行。
相关推荐
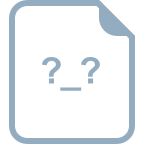















