编写日期时间类,年、月、日、时、分、秒都是数据成员,编写运算符“++”、“- -”、“+”、 “-”、 “>>” 、“<<”的重载函数,在主函数的函数体内中实现使用运算符直接进行日期时间对象的加、减运算。
时间: 2023-11-05 10:12:58 浏览: 20
下面是一个简单的日期时间类的实现,包括上述所需的运算符重载函数:
```C++
#include <iostream>
using namespace std;
class DateTime {
private:
int year, month, day, hour, minute, second;
public:
DateTime(int y = 0, int m = 0, int d = 0, int h = 0, int mi = 0, int s = 0)
: year(y), month(m), day(d), hour(h), minute(mi), second(s) {}
DateTime operator++(int) { // 后置++
DateTime temp(*this);
second++;
if (second == 60) {
second = 0;
minute++;
if (minute == 60) {
minute = 0;
hour++;
if (hour == 24) {
hour = 0;
day++;
if (day > daysInMonth()) {
day = 1;
month++;
if (month > 12) {
month = 1;
year++;
}
}
}
}
}
return temp;
}
DateTime operator--(int) { // 后置--
DateTime temp(*this);
second--;
if (second < 0) {
second = 59;
minute--;
if (minute < 0) {
minute = 59;
hour--;
if (hour < 0) {
hour = 23;
day--;
if (day < 1) {
month--;
if (month < 1) {
year--;
month = 12;
}
day = daysInMonth();
}
}
}
}
return temp;
}
DateTime operator+(const DateTime& dt) const { // 加法
DateTime temp(*this);
temp.second += dt.second;
temp.minute += dt.minute;
temp.hour += dt.hour;
temp.day += dt.day;
temp.month += dt.month;
temp.year += dt.year;
if (temp.second >= 60) {
temp.minute++;
temp.second -= 60;
}
if (temp.minute >= 60) {
temp.hour++;
temp.minute -= 60;
}
if (temp.hour >= 24) {
temp.day++;
temp.hour -= 24;
}
while (temp.day > temp.daysInMonth()) {
temp.day -= temp.daysInMonth();
temp.month++;
if (temp.month > 12) {
temp.year++;
temp.month = 1;
}
}
return temp;
}
DateTime operator-(const DateTime& dt) const { // 减法
DateTime temp(*this);
temp.second -= dt.second;
temp.minute -= dt.minute;
temp.hour -= dt.hour;
temp.day -= dt.day;
temp.month -= dt.month;
temp.year -= dt.year;
if (temp.second < 0) {
temp.minute--;
temp.second += 60;
}
if (temp.minute < 0) {
temp.hour--;
temp.minute += 60;
}
if (temp.hour < 0) {
temp.day--;
temp.hour += 24;
}
while (temp.day < 1) {
temp.month--;
if (temp.month < 1) {
temp.year--;
temp.month = 12;
}
temp.day += temp.daysInMonth();
}
return temp;
}
friend istream& operator>>(istream& in, DateTime& dt) { // 输入流
cout << "Enter year, month, day, hour, minute, second: ";
in >> dt.year >> dt.month >> dt.day >> dt.hour >> dt.minute >> dt.second;
return in;
}
friend ostream& operator<<(ostream& out, const DateTime& dt) { // 输出流
out << dt.year << "-" << dt.month << "-" << dt.day << " " << dt.hour << ":" << dt.minute << ":" << dt.second;
return out;
}
private:
int daysInMonth() const { // 获取当前月份的天数
static const int days[] = { 31,28,31,30,31,30,31,31,30,31,30,31 };
return month == 2 && isLeapYear() ? 29 : days[month - 1];
}
bool isLeapYear() const { // 判断是否为闰年
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
};
int main() {
DateTime dt1, dt2;
cin >> dt1 >> dt2;
cout << "dt1 = " << dt1 << endl;
cout << "dt2 = " << dt2 << endl;
cout << "dt1 + dt2 = " << dt1 + dt2 << endl;
cout << "dt1 - dt2 = " << dt1 - dt2 << endl;
cout << "dt1++ = " << dt1++ << endl;
cout << "dt1 = " << dt1 << endl;
cout << "dt1-- = " << dt1-- << endl;
cout << "dt1 = " << dt1 << endl;
return 0;
}
```
在这个例子中,我们实现了加、减、自增、自减、输入和输出等基本运算符的重载。这里的实现假设年份为四位数,月份为1-12的整数,日期为1-31的整数,小时为0-23的整数,分钟和秒钟为0-59的整数。此外,我们还提供了一些辅助函数来处理日期时间的计算,例如获取当前月份的天数和判断是否为闰年等。
相关推荐
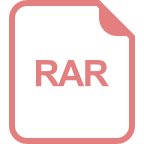
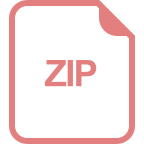





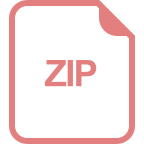
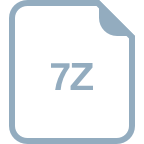
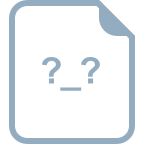
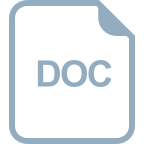
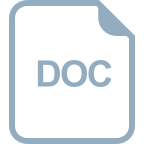
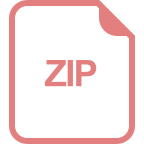
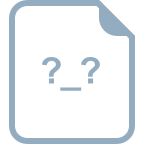
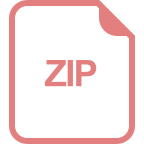
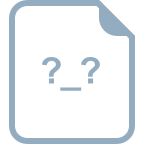