c++题目 编写一个程序,定义一个日期类,含year,month ,day三个整型数据成员,重载运算符==,该函数为非成员函数,功能是判断两个日期对象是否相等。 主函数中测试该功能。
时间: 2023-06-12 10:05:59 浏览: 60
以下是一个简单的实现:
```c++
#include <iostream>
class Date {
public:
Date(int y, int m, int d) : year(y), month(m), day(d) {}
int getYear() const { return year; }
int getMonth() const { return month; }
int getDay() const { return day; }
private:
int year;
int month;
int day;
};
bool operator==(const Date& lhs, const Date& rhs) {
return lhs.getYear() == rhs.getYear() &&
lhs.getMonth() == rhs.getMonth() &&
lhs.getDay() == rhs.getDay();
}
int main() {
Date d1(2021, 9, 1);
Date d2(2021, 9, 2);
Date d3(2021, 9, 1);
std::cout << std::boolalpha;
std::cout << "d1 == d2: " << (d1 == d2) << std::endl;
std::cout << "d1 == d3: " << (d1 == d3) << std::endl;
return 0;
}
```
输出结果:
```
d1 == d2: false
d1 == d3: true
```
在上面的代码中,我们定义了一个 `Date` 类表示日期,其中包含年、月、日三个整型数据成员。然后,我们重载了运算符 `==`,以便能够比较两个日期对象是否相等。最后,我们在主函数中测试了这个功能。
相关推荐
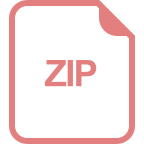
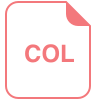













