meanshift图像分割python代码
时间: 2023-07-23 09:16:47 浏览: 112
下面是一份基于skimage库实现的Mean Shift图像分割的Python代码:
```python
from skimage.segmentation import quickshift
from skimage.color import rgb2gray
import matplotlib.pyplot as plt
from skimage.io import imread
# 读取图像
img = imread('image.jpg')
# 转换为灰度图像
img_gray = rgb2gray(img)
# Mean Shift图像分割
segments = quickshift(img_gray, kernel_size=3, max_dist=6, ratio=0.5)
# 显示分割结果
plt.imshow(segments, cmap='gray')
plt.axis('off')
plt.show()
```
其中,`quickshift`函数实现了Mean Shift图像分割,`kernel_size`和`max_dist`分别表示核大小和最大距离,`ratio`是一个平衡参数,可以根据具体情况进行调整。最后通过`imshow`函数显示分割结果。
相关问题
meanshift图像分割python
MeanShift图像分割是一种基于密度估计的非监督学习方法。在Python中,可以使用Scikit-learn库中的MeanShift类来实现MeanShift图像分割。首先,需要导入该类并实例化一个对象,然后调用该对象的fit()方法对图像进行分割。示例代码如下:
```
from sklearn.cluster import MeanShift
import numpy as np
# 实例化MeanShift对象
ms = MeanShift()
# 加载图像并转化为numpy数组
img = ...
img_data = np.array(img)
# 调用fit()方法进行分割
ms.fit(img_data)
# 获取分割后的图像
segmented_img = ms.labels_
```
注意在使用时需要设置的参数如bandwidth, 默认为None,也可以自己设置。
mean-shift图像分割python
以下是使用Mean Shift算法进行图像分割的Python代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.cluster import MeanShift
# 读取图像数据
image = plt.imread('image.jpg')
# 将图像数据转换为二维数组
samples = image.reshape(-1, 3)
# 使用Mean Shift算法进行聚类
bandwidth = 0.1
ms = MeanShift(bandwidth=bandwidth, bin_seeding=True).fit(samples)
# 获取聚类中心
cluster_centers = ms.cluster_centers_
# 根据聚类结果对图像进行分割
segmented_image = cluster_centers[ms.labels_].reshape(image.shape)
# 显示原始图像和分割后的图像
plt.subplot(1, 2, 1)
plt.imshow(image)
plt.title('Original Image')
plt.axis('off')
plt.subplot(1, 2, 2)
plt.imshow(segmented_image)
plt.title('Segmented Image')
plt.axis('off')
plt.show()
```
这段代码首先读取图像数据,并将其转换为二维数组。然后使用Mean Shift算法对图像进行聚类,得到聚类中心。最后根据聚类结果对图像进行分割,并显示原始图像和分割后的图像。
阅读全文
相关推荐
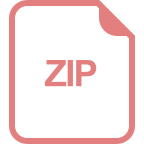
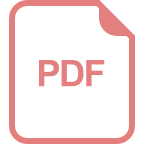





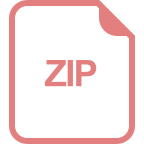
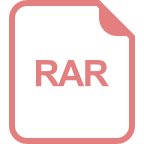
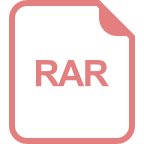


