编写python程序,自定义meanshift方法完成图像分割
时间: 2024-05-10 11:15:22 浏览: 10
好的,下面是一个简单的 Python 程序,用于使用自定义 MeanShift 方法进行图像分割。
首先,需要导入必要的库:
```python
import numpy as np
import cv2
from sklearn.cluster import MeanShift, estimate_bandwidth
import matplotlib.pyplot as plt
```
接下来,读取图像并将其转换为 NumPy 数组:
```python
image = cv2.imread('image.jpg')
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
```
然后,我们需要估计带宽,这将告诉 MeanShift 算法应该在多大的空间内查找像素点:
```python
bandwidth = estimate_bandwidth(image, quantile=0.1, n_samples=100)
```
接下来,我们可以使用 MeanShift 类创建一个估计器,并将其拟合到图像数据中:
```python
ms = MeanShift(bandwidth=bandwidth, bin_seeding=True)
ms.fit(image.reshape(-1, 3))
```
最后,我们可以将像素点分配给它们所属的群集,并在图像上可视化结果:
```python
labels = ms.labels_
cluster_centers = ms.cluster_centers_
segmented_image = np.zeros_like(image)
for i in range(len(image)):
segmented_image[i] = cluster_centers[labels[i]]
plt.figure(figsize=(12, 12))
plt.imshow(segmented_image)
plt.axis('off')
plt.show()
```
完整代码如下:
```python
import numpy as np
import cv2
from sklearn.cluster import MeanShift, estimate_bandwidth
import matplotlib.pyplot as plt
image = cv2.imread('image.jpg')
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
bandwidth = estimate_bandwidth(image, quantile=0.1, n_samples=100)
ms = MeanShift(bandwidth=bandwidth, bin_seeding=True)
ms.fit(image.reshape(-1, 3))
labels = ms.labels_
cluster_centers = ms.cluster_centers_
segmented_image = np.zeros_like(image)
for i in range(len(image)):
segmented_image[i] = cluster_centers[labels[i]]
plt.figure(figsize=(12, 12))
plt.imshow(segmented_image)
plt.axis('off')
plt.show()
```
这个程序可以用于对任何图像进行分割,只需要将 `image.jpg` 替换为所需的图像文件名即可。
相关推荐
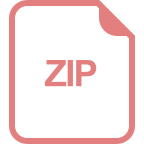
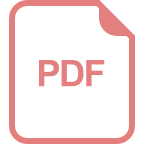
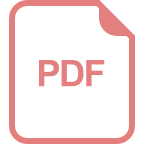
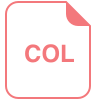
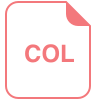
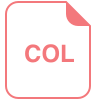
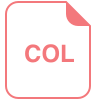
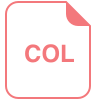









