遍历链表.h文件和.cpp用case输出应该怎么写?
时间: 2024-02-22 20:00:10 浏览: 133
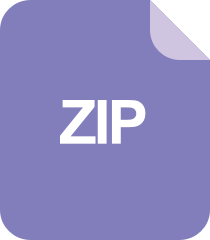
数据结构C++ 单链表的实现 .cpp和.h文件
下面是遍历链表的.h和.cpp文件的代码,可以使用switch case语句输出:
链表头文件 LinkedList.h:
```c++
#ifndef LINKEDLIST_H
#define LINKEDLIST_H
#include <iostream>
struct Node {
int data;
Node* next;
};
class LinkedList {
public:
LinkedList();
~LinkedList();
void insert(int value);
void remove(int value);
void print();
private:
Node* head;
};
#endif // LINKEDLIST_H
```
链表源文件 LinkedList.cpp:
```c++
#include "LinkedList.h"
LinkedList::LinkedList() {
head = nullptr;
}
LinkedList::~LinkedList() {
Node* current = head;
while (current != nullptr) {
Node* next = current->next;
delete current;
current = next;
}
}
void LinkedList::insert(int value) {
Node* newNode = new Node;
newNode->data = value;
newNode->next = head;
head = newNode;
}
void LinkedList::remove(int value) {
Node* current = head;
Node* previous = nullptr;
while (current != nullptr) {
if (current->data == value) {
if (previous == nullptr) {
head = current->next;
} else {
previous->next = current->next;
}
delete current;
return;
}
previous = current;
current = current->next;
}
}
void LinkedList::print() {
Node* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
```
使用switch case输出链表.h和.cpp文件的代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream hFile("LinkedList.h");
std::ifstream cppFile("LinkedList.cpp");
std::string line;
int fileIndex = 0;
while (getline(hFile, line)) {
switch (fileIndex) {
case 0:
std::cout << "// 链表头文件 LinkedList.h:" << std::endl;
break;
case 1:
std::cout << "// 链表源文件 LinkedList.cpp:" << std::endl;
break;
}
std::cout << line << std::endl;
if (line.find("#endif") != std::string::npos) {
++fileIndex;
}
}
while (getline(cppFile, line)) {
switch (fileIndex) {
case 1:
std::cout << "// 链表头文件 LinkedList.h:" << std::endl;
break;
case 2:
std::cout << "// 链表源文件 LinkedList.cpp:" << std::endl;
break;
}
std::cout << line << std::endl;
if (line.find("void LinkedList::print()") != std::string::npos) {
++fileIndex;
}
}
hFile.close();
cppFile.close();
return 0;
}
```
其中,fileIndex变量表示当前正在处理的文件的索引。当读取到`.h`文件的`#endif`预处理指令时,fileIndex加1,表示开始读取`.cpp`文件;当读取到`.cpp`文件的`void LinkedList::print()`函数定义时,fileIndex再次加1,表示`.cpp`文件处理完毕。在switch case语句中,根据fileIndex的值输出对应的注释和代码。
阅读全文
相关推荐
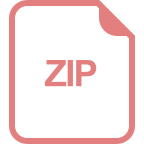
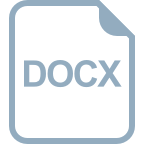
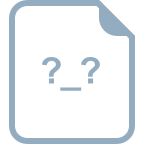
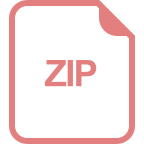
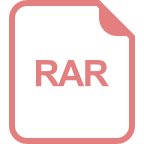
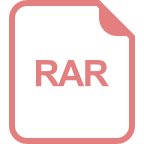
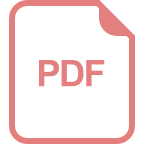
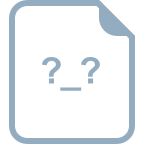
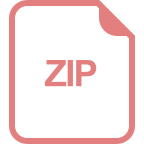
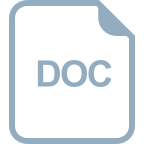
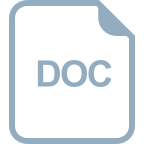
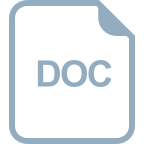





