已知由不具有头结点的单链表表示的线性表中,含有三类字符的数据元素(字母、数字和其他字符),试编写算法构造三个以循环链表表示的线性表,使每个表中只含有同一类的字符,且利用原表中的结点空间,头结点可另辟空间。c++实现
时间: 2024-10-11 11:18:12 浏览: 12
要实现这个功能,我们需要遍历原始链表并根据节点的字符类型将其添加到对应的循环链表中。这里是一个C++的基本示例,展示了如何创建三个循环链表,每个链表只包含一类字符:
```cpp
#include <iostream>
#include <string>
// 定义链表节点结构
struct Node {
char data;
Node* next;
};
class CircularLinkedList {
public:
void addNode(char data) {
if (head == nullptr) {
head = new Node{data, head};
} else {
Node* temp = head;
while (temp->next != head) {
temp = temp->next;
}
temp->next = new Node{data, head};
head->next = temp; // 创建循环
}
}
private:
Node* head;
};
// 主函数
void constructCircularLists(Node* node, CircularLinkedList& alphaList, CircularLinkedList& digitList, CircularLinkedList& otherList) {
Node* current = node;
while (current != nullptr) {
switch (current->data) {
case 'a'...'z':
case 'A'...'Z':
alphaList.addNode(current->data);
break;
case '0'...'9':
digitList.addNode(current->data);
break;
default:
otherList.addNode(current->data);
break;
}
current = current->next;
}
}
int main() {
// 假设我们有一个节点列表node,根据实际数据填充
Node* node = ...;
CircularLinkedList alphaList, digitList, otherList;
constructCircularLists(node, alphaList, digitList, otherList);
// 打印各链表
std::cout << "Alphabetic List: ";
for (Node* temp = alphaList.head; temp != alphaList.head->next; temp = temp->next) {
std::cout << temp->data;
}
std::cout << "\n";
std::cout << "Numeric List: ";
for (Node* temp = digitList.head; temp != digitList.head->next; temp = temp->next) {
std::cout << temp->data;
}
std::cout << "\n";
std::cout << "Other Characters List: ";
for (Node* temp = otherList.head; temp != otherList.head->next; temp = temp->next) {
std::cout << temp->data;
}
std::cout << "\n";
return 0;
}
```
注意,这只是一个基本的示例,实际实现可能需要考虑内存管理和错误处理。同时,由于你提到的是“不具有头结点”的链表,这里的`head`变量可能需要从链表的第一个节点开始。
阅读全文
相关推荐
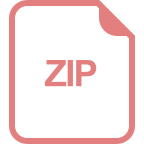
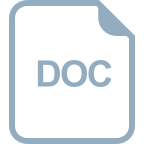













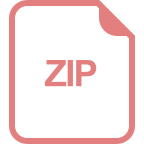