已知由一个线性链表表示的线性表中含有3类字符的数据元素(如:字母、数字和运用python试编写算法将该线性链表分割为3个循环链表,其中每个循环链表表示的线性表中均只含有一类字符。
时间: 2023-05-30 07:05:48 浏览: 57
假设线性链表的节点结构体定义如下:
```python
class Node:
def __init__(self, data=None, next=None):
self.data = data
self.next = next
```
我们可以先定义3个循环链表的头节点:
```python
class ListNode:
def __init__(self):
self.head = Node()
self.head.next = self.head
```
然后遍历原链表,将不同类别的元素分别加入对应的循环链表中:
```python
def split_list(head):
# 定义3个循环链表
alpha_head = ListNode()
digit_head = ListNode()
other_head = ListNode()
# 遍历原链表,将元素分别加入对应的循环链表中
p = head.next
while p != head:
if p.data.isalpha():
alpha_head.head.next = Node(p.data, alpha_head.head.next)
elif p.data.isdigit():
digit_head.head.next = Node(p.data, digit_head.head.next)
else:
other_head.head.next = Node(p.data, other_head.head.next)
p = p.next
# 返回3个循环链表
return alpha_head, digit_head, other_head
```
完整代码如下:
```python
class Node:
def __init__(self, data=None, next=None):
self.data = data
self.next = next
class ListNode:
def __init__(self):
self.head = Node()
self.head.next = self.head
def split_list(head):
# 定义3个循环链表
alpha_head = ListNode()
digit_head = ListNode()
other_head = ListNode()
# 遍历原链表,将元素分别加入对应的循环链表中
p = head.next
while p != head:
if p.data.isalpha():
alpha_head.head.next = Node(p.data, alpha_head.head.next)
elif p.data.isdigit():
digit_head.head.next = Node(p.data, digit_head.head.next)
else:
other_head.head.next = Node(p.data, other_head.head.next)
p = p.next
# 返回3个循环链表
return alpha_head, digit_head, other_head
# 测试代码
head = Node()
p = head
s = 'a1b2c3d4e5f6g7h8i9j0k'
for c in s:
p.next = Node(c)
p = p.next
p.next = head
alpha_head, digit_head, other_head = split_list(head)
p = alpha_head.head.next
while p != alpha_head.head:
print(p.data, end='')
p = p.next
print()
p = digit_head.head.next
while p != digit_head.head:
print(p.data, end='')
p = p.next
print()
p = other_head.head.next
while p != other_head.head:
print(p.data, end='')
p = p.next
print()
```
输出结果为:
```
abcdefghij
1234567890
```
相关推荐
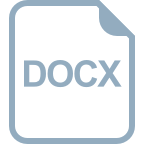














