编写C语言程序,模拟实现首次/最佳/最坏适应算法的内存块分配和回收,要求使用数组,每次分配和回收后显示出空闲分区和已分配分区的情况。假设初始状态下,可用的内存空间为640KB。
时间: 2024-12-14 11:20:56 浏览: 58
在C语言中,我们可以使用结构体表示内存块,数组存储所有的内存块信息,以及一些辅助函数来模拟首次适应、最佳适应和最坏适应算法。以下是简化的实现步骤:
1. 定义内存块结构体:
```c
typedef struct {
int size; // 内存块大小
int status; // 状态:0 - 空闲,1 - 已分配
} MemoryBlock;
```
2. 初始化内存池:
```c
#define TOTAL_MEMORY 65536 // 640KB (实际内存大小)
MemoryBlock memory[TOTAL_MEMORY] = {0}; // 全部初始化为0,代表空闲状态
int freeBlocks = TOTAL_MEMORY; // 记录空闲分区数量
```
3. 首次适应算法:
```c
void first_fit(int request) {
for (int i = 0; i < freeBlocks; i++) {
if (memory[i].size >= request) {
memory[i].status = 1; // 分配给请求
printf("Allocated %d bytes to block %d\n", request, i);
freeBlocks--;
break;
}
}
}
```
4. 最佳适应算法(找到最小的连续空闲区):
```c
void best_fit(int request) {
for (int i = 0; i < freeBlocks; i++) {
if (i + request <= TOTAL_MEMORY && memory[i:i+request][0].status == 0) {
for (int j = i; j < i + request; j++)
memory[j].status = 1;
printf("Allocated %d bytes starting at block %d\n", request, i);
freeBlocks -= request;
break;
}
}
}
```
5. 最坏适应算法(找到最大的连续空闲区):
```c
void worst_fit(int request) {
int maxFreeIndex = 0;
int maxSize = 0;
for (int i = 0; i < freeBlocks; i++) {
if (memory[i:].size > maxSize) {
maxFreeIndex = i;
maxSize = memory[i:].size;
}
}
if (maxSize >= request) {
// 分配给请求
for (int i = maxFreeIndex; i < maxFreeIndex + request; i++)
memory[i].status = 1;
printf("Allocated %d bytes starting at block %d\n", request, maxFreeIndex);
freeBlocks -= request;
} else {
printf("Not enough space for the request.\n");
}
}
```
6. 内存回收函数:
```c
void recycle(int deallocationIndex) {
memory[deallocationIndex].status = 0; // 回收内存
freeBlocks++; // 空闲分区数量加一
printf("Recycled block %d\n", deallocationIndex);
}
```
7. 主循环示例:
```c
int main() {
int request;
while (scanf("%d", &request) != EOF) {
switch (yourChoice) { // 根据需求选择对应算法
case 1: first_fit(request); break;
case 2: best_fit(request); break;
case 3: worst_fit(request); break;
// 添加释放内存操作
}
}
return 0;
}
```
在这个例子中,你需要添加一个用户输入的选择`yourChoice`,以便根据选择调用相应的内存分配算法。同时,别忘了处理内存回收情况。
阅读全文
相关推荐
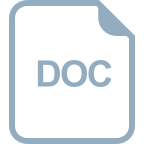
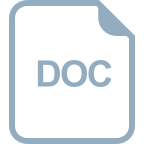
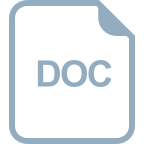

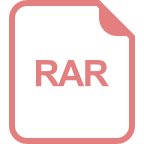
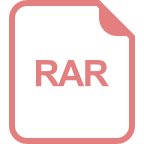
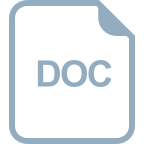
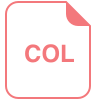
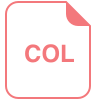
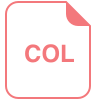
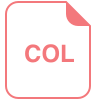
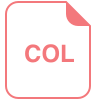
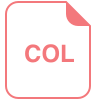
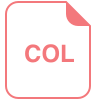
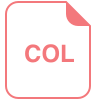
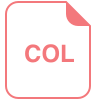
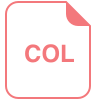
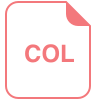
