链表中节点值为k的元素与尾节点交换位置请使用C语言实现
时间: 2024-09-07 11:01:55 浏览: 41
首先,我们定义链表节点的数据结构,然后编写一个函数来实现交换链表中值为k的元素与尾节点的位置。这里需要注意的是,链表的尾节点指的是最后一个节点,而值为k的元素可能不是唯一的。以下是一个可能的实现方法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct ListNode {
int val;
struct ListNode *next;
};
// 创建一个新的链表节点
struct ListNode* createNode(int val) {
struct ListNode* newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->val = val;
newNode->next = NULL;
return newNode;
}
// 交换链表中值为k的节点与尾节点的位置
struct ListNode* swapNodes(struct ListNode* head, int k) {
if (head == NULL || head->next == NULL || k <= 0) {
return head;
}
struct ListNode *nodeToSwap = NULL, *prev = NULL, *current = head;
struct ListNode *tail = NULL;
// 寻找第k个节点
while (current != NULL && k > 1) {
prev = current;
current = current->next;
k--;
}
if (current == NULL) {
return head; // k大于链表长度,不进行交换
}
nodeToSwap = current;
// 找到尾节点
while (current->next != NULL) {
current = current->next;
tail = current;
}
// 交换值为k的节点和尾节点的位置
if (prev == NULL) { // 如果k节点是头节点
head = nodeToSwap->next;
nodeToSwap->next = tail->next;
tail->next = nodeToSwap;
} else {
prev->next = nodeToSwap->next;
nodeToSwap->next = tail->next;
tail->next = nodeToSwap;
}
return head;
}
// 打印链表
void printList(struct ListNode* head) {
struct ListNode* temp = head;
while (temp != NULL) {
printf("%d ", temp->val);
temp = temp->next;
}
printf("\n");
}
// 释放链表内存
void freeList(struct ListNode* head) {
struct ListNode* temp;
while (head != NULL) {
temp = head;
head = head->next;
free(temp);
}
}
// 主函数
int main() {
struct ListNode* head = createNode(1);
head->next = createNode(2);
head->next->next = createNode(3);
head->next->next->next = createNode(4);
head->next->next->next->next = createNode(5);
printf("Original list: ");
printList(head);
head = swapNodes(head, 3);
printf("List after swapping: ");
printList(head);
freeList(head);
return 0;
}
```
在这个实现中,我们首先遍历链表找到第k个节点和尾节点。如果k节点是头节点,我们需要特殊处理,否则我们将把k节点与尾节点进行位置交换。最后,我们打印出修改后的链表并释放分配的内存。
阅读全文
相关推荐
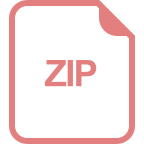
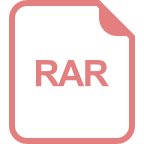
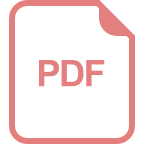
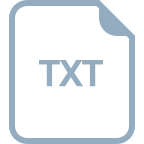
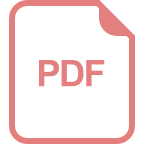
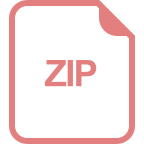
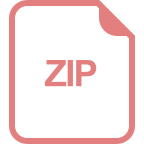
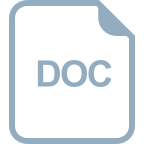
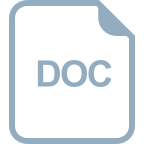
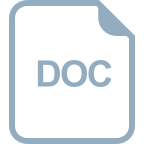
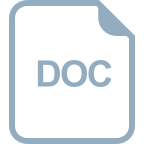
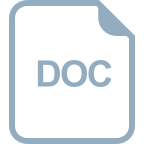
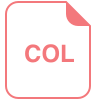
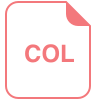




