python打地鼠小游戏代码
时间: 2024-12-07 16:10:06 浏览: 23
Python打地鼠小游戏通常是一个基础的控制台游戏,使用了基本的游戏循环、随机事件和用户输入处理。以下是一个简单的例子,使用了`random`库来模拟地鼠出现和消失,以及`time`库让游戏暂停:
```python
import random
import time
class Mole:
def __init__(self):
self.position = random.randint(0, 4)
def move(self):
self.position = (self.position + 1) % 5 # 地鼠移动到下一个位置
def play():
mole = Mole()
print("游戏开始!按任意键显示地鼠...")
input() # 等待玩家按下任意键
print(f"你在第{mole.position}号格发现了地鼠!")
while True:
mole.move() # 地鼠随机移动
time.sleep(1) # 模拟地鼠隐藏过程,暂停1秒
print("地鼠消失了...")
play()
```
相关问题
python打地鼠游戏完整代码
以下是一个简单的Python打地鼠游戏的完整代码示例:
```python
import pygame
import random
# 初始化游戏
pygame.init()
# 设置游戏窗口大小
window_width = 800
window_height = 600
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption("打地鼠游戏")
# 加载背景图片
background_image = pygame.image.load("background.jpg")
# 加载地鼠图片
mole_image = pygame.image.load("mole.png")
# 设置地鼠的初始位置
mole_x = random.randint(0, window_width - mole_image.get_width())
mole_y = random.randint(0, window_height - mole_image.get_height())
# 游戏主循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 绘制背景
window.blit(background_image, (0, 0))
# 绘制地鼠
window.blit(mole_image, (mole_x, mole_y))
# 更新屏幕
pygame.display.flip()
# 退出游戏
pygame.quit()
```
python打地鼠游戏代码
以下是一个简单的 Python 打地鼠游戏代码:
```python
import pygame
import random
# 初始化
pygame.init()
# 游戏窗口大小
width = 640
height = 480
# 颜色定义
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
# 创建游戏窗口
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("打地鼠")
# 创建游戏时钟
clock = pygame.time.Clock()
# 地鼠类
class Mole(pygame.sprite.Sprite):
def __init__(self):
pygame.sprite.Sprite.__init__(self)
self.image = pygame.Surface((40, 40))
self.image.fill(red)
self.rect = self.image.get_rect()
self.rect.center = (random.randint(50, width-50), random.randint(50, height-50))
def update(self):
self.rect.center = (random.randint(50, width-50), random.randint(50, height-50))
# 所有精灵组
all_sprites = pygame.sprite.Group()
# 地鼠组
mole_group = pygame.sprite.Group()
# 创建地鼠
for i in range(10):
mole = Mole()
all_sprites.add(mole)
mole_group.add(mole)
# 游戏循环
score = 0
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
for mole in mole_group:
if mole.rect.collidepoint(event.pos):
score += 1
mole.kill()
# 清屏
screen.fill(white)
# 更新所有精灵
all_sprites.update()
# 绘制所有精灵
all_sprites.draw(screen)
# 绘制得分
font = pygame.font.Font(None, 36)
text = font.render("Score: " + str(score), True, black)
screen.blit(text, (10, 10))
# 刷新屏幕
pygame.display.flip()
# 控制游戏帧率
clock.tick(10)
# 退出游戏
pygame.quit()
```
这个游戏很简单,就是在屏幕上随机出现地鼠,玩家通过鼠标点击地鼠来得分。这个示例代码使用 Pygame 游戏框架来实现游戏窗口、精灵的管理、事件处理等功能。
阅读全文
相关推荐
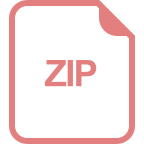
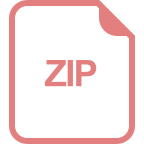
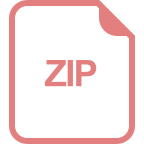


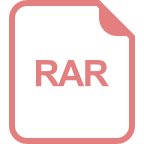
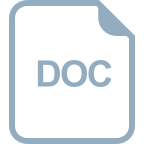







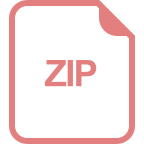
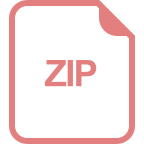