esp8266在ap模式手机控制led编程
时间: 2023-11-24 13:02:45 浏览: 234
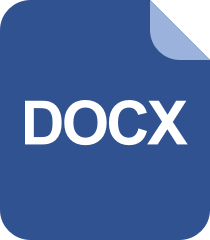
用手机控制Arduino上的LED灯(使用ESP8266模块的AT指令方式).docx
esp8266是一种低成本、高性能的wifi模块,可以用来实现手机远程控制led灯的编程。首先,我们需要将esp8266模块设置成AP模式,这样手机才能连接到它的热点上。接着,我们需要在esp8266上编写一个简单的web服务器程序,使手机可以通过浏览器访问该服务器,进而远程控制led灯的开关。
在esp8266的程序中,我们需要使用一些基本的代码和指令,如配置wifi、设置端口、建立http服务等。当手机连接到esp8266的热点后,打开浏览器并输入相应的IP地址和端口号,就可以通过网页控制led的亮灭。通过手机上的操作,我们可以发送指令到esp8266,从而实现led的开关控制。这样,无论用户在何处,只要手机和esp8266都连接到网络上,就可以实现对led的远程控制。
在编程的过程中,我们需要考虑多种情况,如网络连接的稳定性、用户指令的合法性等。同时,为了提高用户体验,我们还可以为led的控制界面添加一些个性化的设计,如图形化按钮、实时状态显示等功能。通过这种方法,我们就可以实现手机对led的远程控制,为用户提供更加便利的体验。
阅读全文
相关推荐
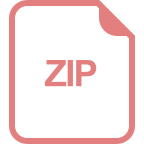
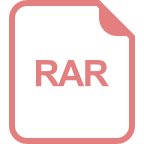

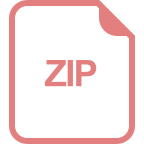
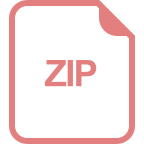
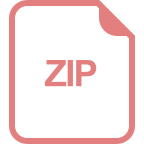
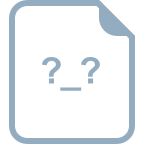
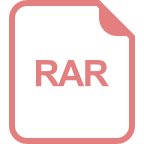
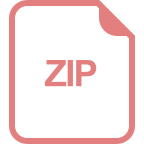
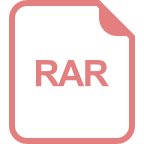
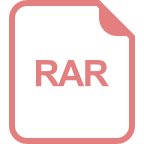
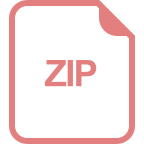
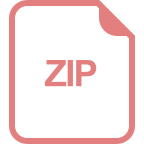
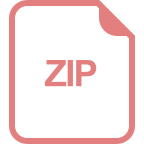
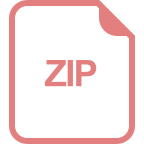
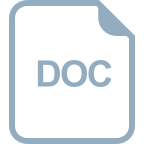
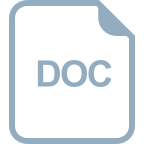