比如有很多excel文件在一个文件夹内,它们的格式都相同,我想把每个文件中的同一个位置的数据一起提出来,存储在一个新的excle表格中,这个该怎么实现啊?
时间: 2024-11-18 15:18:18 浏览: 14
你可以通过Python的pandas库来实现这样的数据提取操作。以下是步骤:
1. **导入必要的库**:
首先需要安装`pandas`库,如果还没有安装,可以使用pip安装:
```
pip install pandas
```
2. **读取Excel文件**:
使用`pandas`的`read_excel`函数逐个读取文件夹中的Excel文件,并将数据存入一个列表或字典结构中,比如DataFrame对象的列表。
```python
import os
import pandas as pd
# 获取文件夹路径
folder_path = "your_folder_path"
files = [os.path.join(folder_path, f) for f in os.listdir(folder_path) if f.endswith('.xlsx')]
data_frames = []
for file in files:
df = pd.read_excel(file)
data_frames.append(df)
```
3. **处理和合并数据**:
对于每个文件中同一位置的数据,你可以选择直接提取到一个新的DataFrame,或者使用相同的索引位置合并所有DataFrame。这里假设你要提取的是每行的某一列。
```python
target_column = 'your_target_column' # 替换为你想要提取的列名
common_index = data_frames[0].index # 假设所有文件有相同的索引
result_df = pd.DataFrame(index=common_index)
for df in data_frames:
result_df[target_column] = df.loc[df.index, target_column]
```
4. **保存结果**:
最后,将处理后的数据保存到新的Excel文件中:
```python
output_file = "output.xlsx"
result_df.to_excel(output_file, index=True)
```
阅读全文
相关推荐
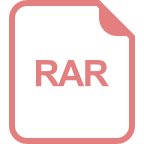
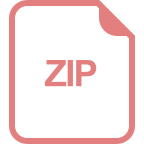
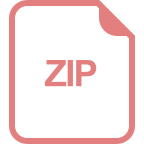
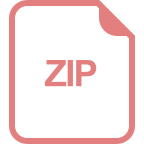
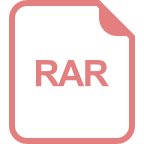
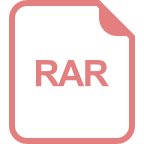
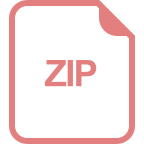
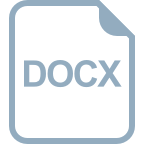
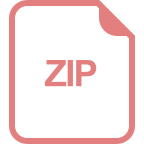
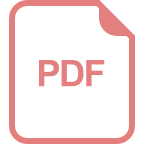
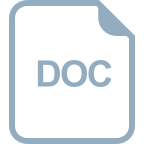
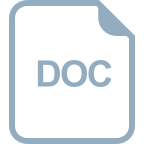






