用c语言写一篇高斯滤波法处理bmp图像的代码
时间: 2024-09-09 19:07:41 浏览: 67
高斯滤波是一种图像处理中常用的平滑技术,它可以减少图像噪声和细节,常用于图像模糊处理。下面是使用C语言进行高斯滤波处理BMP图像的一个简单示例代码。请注意,这里假设你已经有了处理BMP文件的基础知识,比如如何读取和写入BMP格式的图像数据。
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define PI 3.14159265
#define GAUSSIAN_RADIUS 2 // 高斯核的半径
#define GAUSSIAN_GRAYSCALE 15 // 高斯核的中间值,用于归一化
// 函数声明
void calculateGaussianKernel(float *kernel, int radius);
void applyGaussianBlur(unsigned char *src, unsigned char *dst, int width, int height, float *kernel);
// 主函数
int main() {
// 假设 BMP 图像数据已经加载到这个数组中
unsigned char *imgData;
// 假设我们已经有了图像的宽度和高度
int width;
int height;
// ... 代码加载 BMP 图像数据到 imgData ...
// 为高斯核分配内存
float *gaussianKernel = (float *)malloc((GAUSSIAN_RADIUS * 2 + 1) * sizeof(float));
// 计算高斯核
calculateGaussianKernel(gaussianKernel, GAUSSIAN_RADIUS);
// 分配内存给新的图像数据,用于存放处理后的图像
unsigned char *newImgData = (unsigned char *)malloc(width * height * sizeof(unsigned char));
// 应用高斯滤波
applyGaussianBlur(imgData, newImgData, width, height, gaussianKernel);
// ... 代码保存处理后的图像 ...
// 清理
free(gaussianKernel);
free(newImgData);
// ... 代码释放 imgData ...
return 0;
}
// 计算高斯核
void calculateGaussianKernel(float *kernel, int radius) {
float sum = 0.0;
int i, j;
for (i = -radius; i <= radius; i++) {
for (j = -radius; j <= radius; j++) {
kernel[(i + radius) * (2 * radius + 1) + (j + radius)] =
exp(-(i * i + j * j) / (2.0 * GAUSSIAN_GRAYSCALE * GAUSSIAN_GRAYSCALE)) / (2.0 * PI * GAUSSIAN_GRAYSCALE * GAUSSIAN_GRAYSCALE);
sum += kernel[(i + radius) * (2 * radius + 1) + (j + radius)];
}
}
// 归一化高斯核
for (i = 0; i < (2 * radius + 1) * (2 * radius + 1); i++) {
kernel[i] /= sum;
}
}
// 应用高斯滤波
void applyGaussianBlur(unsigned char *src, unsigned char *dst, int width, int height, float *kernel) {
int radius = (int)sqrt((2 * radius + 1) * (2 * radius + 1)) / 2;
int x, y, i, j;
int sum, k;
for (y = 0; y < height; y++) {
for (x = 0; x < width; x++) {
sum = 0;
for (i = -radius; i <= radius; i++) {
for (j = -radius; j <= radius; j++) {
int newX = x + j;
int newY = y + i;
if (newX >= 0 && newX < width && newY >= 0 && newY < height) {
sum += src[newY * width + newX] * kernel[(i + radius) * (2 * radius + 1) + (j + radius)];
}
}
}
dst[y * width + x] = sum / (256 * (2 * radius + 1) * (2 * radius + 1));
}
}
}
```
请注意,这段代码是一个非常简化的示例,它没有包括读取和写入BMP图像文件的完整代码。你需要使用适当的方法来加载BMP图像数据到`imgData`,并从`newImgData`保存回文件。此外,你还需要根据实际情况调整高斯核的大小和权重。
阅读全文
相关推荐
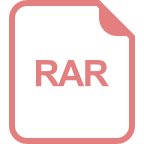
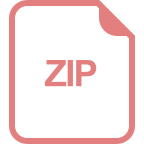
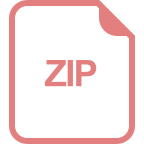
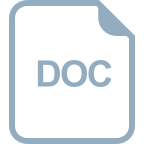
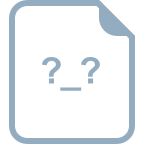
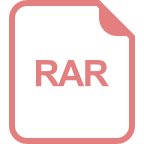


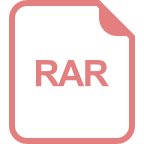
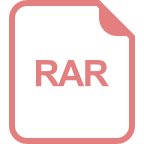
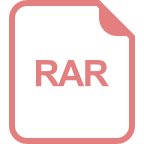
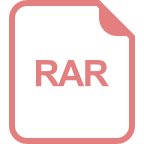
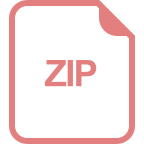
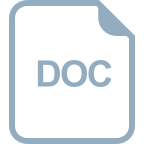
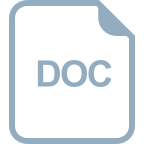
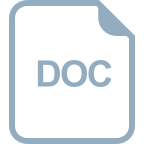
