用C语言写一个灰度图像的高斯滤波函数,并说明
时间: 2024-02-20 18:59:54 浏览: 30
好的,下面是一个用C语言实现的灰度图像高斯滤波函数的代码,并附有详细的注释说明。
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define PI 3.14159265358979323846
// 定义高斯滤波器的大小和标准差
#define FILTER_SIZE 5
#define SIGMA 1.0
// 计算高斯核函数的值
double gaussian(double x, double y, double sigma)
{
double coefficient = 1.0 / (2.0 * PI * sigma * sigma);
double exponent = -(x * x + y * y) / (2.0 * sigma * sigma);
return coefficient * exp(exponent);
}
// 图像高斯滤波函数
void gaussian_filter(unsigned char *image, int width, int height)
{
// 定义高斯核
double kernel[FILTER_SIZE][FILTER_SIZE];
double sum = 0.0;
int i, j, k, l;
// 计算高斯核
for (i = -FILTER_SIZE / 2; i <= FILTER_SIZE / 2; i++)
{
for (j = -FILTER_SIZE / 2; j <= FILTER_SIZE / 2; j++)
{
kernel[i + FILTER_SIZE / 2][j + FILTER_SIZE / 2] = gaussian(i, j, SIGMA);
sum += kernel[i + FILTER_SIZE / 2][j + FILTER_SIZE / 2];
}
}
// 归一化高斯核
for (i = 0; i < FILTER_SIZE; i++)
{
for (j = 0; j < FILTER_SIZE; j++)
{
kernel[i][j] /= sum;
}
}
// 复制原图像
unsigned char *copy = (unsigned char *) malloc(sizeof(unsigned char) * width * height);
for (i = 0; i < width * height; i++)
{
copy[i] = image[i];
}
// 对每个像素进行高斯滤波
for (i = FILTER_SIZE / 2; i < height - FILTER_SIZE / 2; i++)
{
for (j = FILTER_SIZE / 2; j < width - FILTER_SIZE / 2; j++)
{
double sum = 0.0;
for (k = -FILTER_SIZE / 2; k <= FILTER_SIZE / 2; k++)
{
for (l = -FILTER_SIZE / 2; l <= FILTER_SIZE / 2; l++)
{
sum += kernel[k + FILTER_SIZE / 2][l + FILTER_SIZE / 2] * copy[(i + k) * width + j + l];
}
}
image[i * width + j] = (unsigned char) sum;
}
}
// 释放内存
free(copy);
}
```
此函数接收三个参数:
- `image`:需要进行高斯滤波的图像数据,类型为 `unsigned char *`,存储顺序为行优先
- `width`:图像的宽度,类型为 `int`
- `height`:图像的高度,类型为 `int`
函数中使用了一个 `gaussian` 函数来计算高斯核函数的值,并使用了一个二维数组 `kernel` 来存储高斯核。函数首先计算高斯核,并将其归一化后存储在 `kernel` 数组中。然后复制原图像数据,并对每个像素进行高斯滤波,最后将滤波后的图像数据存储回 `image` 数组中。
需要注意的是,实现高斯滤波函数时需要考虑边界问题。此函数使用了一个简单的方法来处理边界,在计算某个像素的值时,只使用其周围的像素,而不考虑超出图像边界的像素。这种方法可能会在图像边缘产生一些不自然的效果,因此在实际应用中需要针对具体情况进行调整。
相关推荐
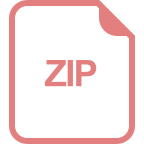









