银行家算法python语言
时间: 2023-12-08 08:39:18 浏览: 37
银行家算法是一种避免死锁的算法,它通过动态地分配资源来避免死锁的发生。下面是一个简单的银行家算法的Python实现:
```python
# 定义银行家算法类
class BankerAlgorithm:
def __init__(self, available, max, allocation):
self.available = available # 可用资源
self.max = max # 最大需求矩阵
self.allocation = allocation # 已分配矩阵
self.need = self.max - self.allocation # 需求矩阵
self.safe_sequence = [] # 安全序列
# 检查当前状态是否安全
def is_safe(self):
work = self.available.copy()
finish = [False] * len(self.max)
while False in finish:
found = False
for i in range(len(self.max)):
if not finish[i] and all(self.need[i] <= work):
work += self.allocation[i]
finish[i] = True
self.safe_sequence.append(i)
found = True
if not found:
return False
return True
# 请求资源
def request(self, request):
if all(request <= self.need) and all(request <= self.available):
self.available -= request
self.allocation += request
self.need = self.max - self.allocation
if self.is_safe():
return True
else:
self.available += request
self.allocation -= request
self.need = self.max - self.allocation
return False
else:
return False
# 释放资源
def release(self, release):
self.available += release
self.allocation -= release
self.need = self.max - self.allocation
# 测试
if __name__ == '__main__':
available = [3, 3, 2]
max = [[7, 5, 3], [3, 2, 2], [9, 0, 2]]
allocation = [[0, 1, 0], [2, 0, 0], [3, 0, 2]]
banker = BankerAlgorithm(available, max, allocation)
request = [1, 0, 2]
if banker.request(request):
print("Request granted")
print("Safe sequence:", banker.safe_sequence)
else:
print("Request denied")
```
以上代码实现了银行家算法的主要功能,包括检查当前状态是否安全、请求资源和释放资源。在测试部分,我们定义了一个初始状态,然后请求一些资源,如果请求被允许,就输出安全序列,否则输出请求被拒绝。
相关推荐
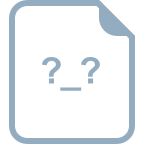













